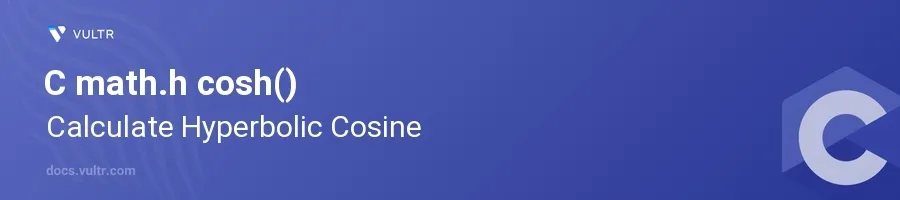
Introduction
The cosh()
function in C, part of the <math.h>
header, computes the hyperbolic cosine of a given angle measured in radians. Hyperbolic cosine is an important mathematical function frequently used in various scientific calculations, including physics and engineering, to describe the shape of a hanging cable or in the theory of relativity.
In this article, you will learn how to accurately calculate the hyperbolic cosine of an angle using the cosh()
function in C. Explore how this function operates with different data types and its practical application within program scenarios, focusing on precision and potential use cases.
Calculating Hyperbolic Cosine with cosh()
Basic Usage of cosh()
Include the
<math.h>
header file in your C program to use thecosh()
function.Declare a variable to store the angle in radians.
Call the
cosh()
function, and store the result.c#include <stdio.h> #include <math.h> int main() { double angle = 1.0; // angle in radians double result = cosh(angle); printf("The hyperbolic cosine of %f is %f\n", angle, result); return 0; }
This code snippet calculates the hyperbolic cosine of
1.0
radians. Thecosh()
function computes the result, which is then printed.
Dealing with Different Angles
Test
cosh()
with various angles, including negatives and values greater than2π
(approximately 6.283).Display results to observe the function's behavior under different conditions.
c#include <stdio.h> #include <math.h> int main() { double angles[] = {0, -1, 3.14159, 6.283}; int i; for(i = 0; i < 4; i++) { double angle = angles[i]; double result = cosh(angle); printf("The hyperbolic cosine of %f is %f\n", angle, result); } return 0; }
This example iterates over an array of different angles and calculates the hyperbolic cosine for each. Notice how the
cosh()
function handles negative values and angles greater than2π
.
Practical Application Example
Apply
cosh()
to model real-world phenomena, such as simulating a hanging cable curve.Use appropriate data and simulate the scenario.
c#include <stdio.h> #include <math.h> void displayHangingCableShape(double length, int points) { double increment = length / points; double x; for(x = 0; x <= length; x += increment) { double height = cosh(x - length / 2); printf("Height at %f: %f\n", x, height); } } int main() { displayHangingCableShape(10.0, 100); return 0; }
In this code,
cosh()
models the curvature of a hanging cable across a specified length. The function generates height values along the cable, yielding a realistic curve depiction.
Conclusion
Utilizing the cosh()
function in C enables precise calculations of the hyperbolic cosine of angles in radians, providing essential capabilities for mathematical and scientific computations. By incorporating this function into diverse programming scenarios, from simple angle calculations to complex physical simulations, enhance the accuracy and reliability of your applications. Tailor the use of cosh()
to meet specific needs, ensuring your mathematical computations are both robust and efficient.
No comments yet.