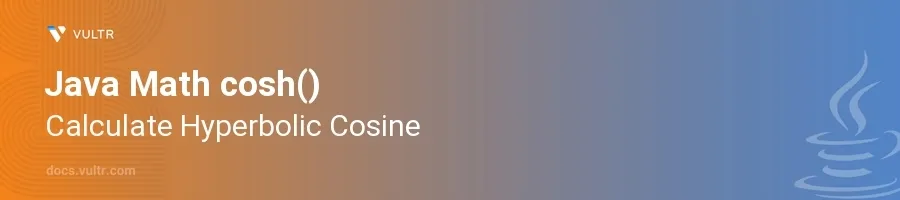
Introduction
The Math.cosh()
method in Java computes the hyperbolic cosine of a double value, providing essential functionality when working with hyperbolic trigonometric functions. It is often used in various scientific, engineering, and mathematical computations, particularly those involving hyperbolic shapes and waves.
In this article, you will learn how to use the Math.cosh()
method in Java effectively. Explore practical examples that illustrate how to calculate the hyperbolic cosine values and apply this method in different programming scenarios to enhance your numerical computation capabilities.
Utilizing Math.cosh() in Java
Calculate the Hyperbolic Cosine of a Number
Make sure to pass a parameter of type
double
to theMath.cosh()
method.Assign the result to a variable and print it for verification.
javadouble value = 1.0; double result = Math.cosh(value); System.out.println("Hyperbolic Cosine of " + value + " is: " + result);
This code calculates the hyperbolic cosine of
1.0
. The method returns a result, which is then printed to the console.
Observing the Hyperbolic Cosine of Zero and Large Values
Understand that the hyperbolic cosine of zero is defined and should return
1.0
.Evaluate
Math.cosh(0)
and print the result.Test
Math.cosh()
with a larger value to observe exponential growth.javadouble zeroValue = 0; double largeValue = 10; System.out.println("Hyperbolic Cosine of 0 is: " + Math.cosh(zeroValue)); System.out.println("Hyperbolic Cosine of 10 is: " + Math.cosh(largeValue));
The first print statement should display
1.0
asMath.cosh(0)
results in1.0
. The second statement demonstrates how the hyperbolic cosine grows exponentially as the input becomes large.
Comparing cosh() with Other Trigonometric Functions
Calculate the hyperbolic cosine, cosine, and exponential equivalents to compare them.
Display the results for a single value to see the differences clearly.
javadouble compareValue = 3; double hyperbolicResult = Math.cosh(compareValue); double cosineResult = Math.cos(compareValue); double exponentialResult = (Math.exp(compareValue) + Math.exp(-compareValue)) / 2; System.out.println("Cosh(" + compareValue + "): " + hyperbolicResult); System.out.println("Cos(" + compareValue + "): " + cosineResult); System.out.println("Exponential Calculation: " + exponentialResult);
This code snippet shows the results of the hyperbolic cosine, the standard cosine, and a manually calculated exponential form. It serves to illustrate the difference in behavior between these functions.
Conclusion
The Math.cosh()
function in Java is invaluable for calculating the hyperbolic cosine, crucial in many advanced mathematics, physics, and engineering calculations. Whether analyzing wave forms, exploring exponential growth patterns, or merely comparing different trigonometric functions, understanding how to use this method enhances the precision and capabilities of your Java programs. By implementing the examples provided, you ensure your calculations remain accurate and your programming skills stay sharp.
No comments yet.