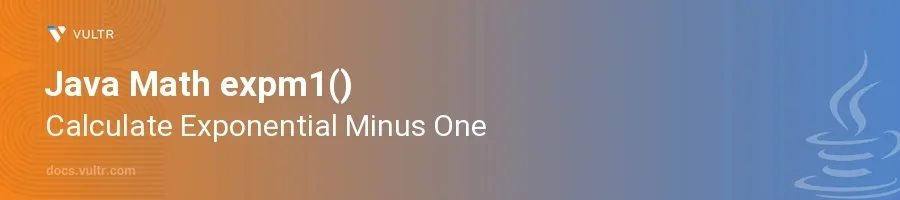
Introduction
The expm1()
method in Java belongs to the Math
class and is utilized to compute (e^x) - 1
, where e
is the base of natural logarithms, approximately equal to 2.718. This method provides higher precision, especially for small values of x
. It's particularly beneficial in fields involving mathematical and scientific calculations where small numeric differences are critical.
In this article, you will learn how to accurately use the Math.expm1()
method in Java across a variety of use cases. Mastering this function can help optimize the precision in your calculations and enhance the overall performance of your applications involving exponential computations in Java. Understanding how to handle exponential in Java efficiently ensures better numerical accuracy and improved application reliability.
Understanding the expm1() Method
Basic Usage of expm1()
Understand the purpose:
expm1()
computes(e^x) - 1
precisely, even whenx
is very small. This precision is crucial in avoiding the loss of significance that can occur with small x values, if simply usingMath.exp(x) - 1
.
Syntax and return:
- The method signatures are as follows:
javapublic static double expm1(double x)
- It returns the value
(e^x) - 1
as adouble
.
Sample calculation:
- Compute
(e^1) - 1
usingexpm1()
, expecting a result that closely approachese - 1
.
javadouble x = 1.0; double result = Math.expm1(x); System.out.println("expm1(1.0) = " + result);
This computes the value of
(e^1) - 1
and outputs it. The result should be close to 1.718, depending on the precision of the floating-point arithmetic of the JVM.- Compute
Comparing expm1() With exp()
Understand the value of using
expm1()
overMath.exp() - 1
:- For very small values of
x
,Math.exp(x) - 1
can yield inaccurate results due to the limitations of floating-point arithmetic.
- For very small values of
Perform a comparison:
- Calculate
(e^0.001) - 1
using both methods and observe the difference.
javadouble smallX = 0.001; double expResult = Math.exp(smallX) - 1; double expm1Result = Math.expm1(smallX); System.out.println("Math.exp(0.001) - 1 = " + expResult); System.out.println("Math.expm1(0.001) = " + expm1Result);
This example demonstrates that
expm1(smallX)
is likely to provide a more accurate result compared toMath.exp(smallX) - 1
whenx
is small. This is due to better handling of precision in theexpm1()
method.- Calculate
Use Cases of expm1()
Financial Calculations
Apply
expm1()
for continuously compounded interest:- Use this method in scenarios involving continuous compounding where small rates are compounded over many periods.
Example calculation:
- Calculate the future value of an investment under continuous compounding.
javadouble principal = 1000.0; // Principal amount in dollars double annualRate = 0.05; // Annual interest rate double time = 10; // Time period in years double amount = principal * Math.expm1(annualRate * time); System.out.println("Future value: " + amount);
This calculates the amount after 10 years of continuous compounding at a 5% rate.
Scientific Modeling
Use
expm1()
in population growth models:- The method can be integral in models where exponential growth factors into the equation, like in population or chemical reaction studies.
Example for a growth model:
- Estimate the population growth over a year when the growth rate is very small.
javadouble initialPop = 1500; // Initial population double growthRate = 0.014; // 1.4% annual growth rate double newPopulation = initialPop * Math.expm1(growthRate); System.out.println("Expected population after one year: " + newPopulation);
This calculates and projects the population after one year based on a 1.4% growth rate.
Conclusion
The Math.expm1()
method in Java is a crucial tool for ensuring precision in calculations involving exponential functions, especially when dealing with small values of x
. Use this method to maintain accuracy and prevent data loss in various scientific, engineering, and financial applications. By integrating expm1()
into your Java applications, maintain high precision in computational tasks which is vital for producing reliable and accurate results.
No comments yet.