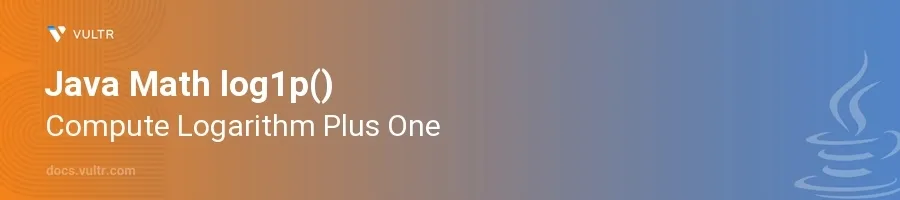
Introduction
The log1p()
method in Java belongs to the Math
class and is designed to compute the natural logarithm of (1 + x)
, where x
is a real number. This function is particularly useful in numerical computations where precision losses would occur if 1 + x
is very close to 1
, specifically for very small values of x
. It helps maintain higher precision compared to direct calculations involving Math.log(1 + x)
.
In this article, you will learn how to utilize the log1p()
method in Java. Explore how this method functions with different types of values, its advantages in computational accuracy, and some practical examples where it can be effectively applied in programming scenarios.
Understanding log1p()
Basic Usage of log1p()
Start with the basic syntax of the
log1p()
function in Java.Explore its return behavior with a simple example.
javadouble result = Math.log1p(2.0); System.out.println("Logarithm of 1 + 2.0 is: " + result);
This code computes the natural logarithm of 3.0 (
1 + 2.0
) and prints the result. Here,log1p(2.0)
efficiently calculates the value without precision losses.
Handling Special Values
Understand how
log1p()
handles different types of special input values, such as negative numbers and zero.Demonstrate with examples.
javaSystem.out.println("Logarithm of 1.0: " + Math.log1p(0)); System.out.println("Logarithm when x is -1: " + Math.log1p(-1)); System.out.println("Logarithm for very small positive close to zero: " + Math.log1p(1e-10));
- The first print statement outputs the logarithm of 1.0, which is
0
, sinceMath.log1p(0)
islog(1)
. - The second statement handles the special case where
x
is-1
, outputting negative infinity, as the logarithm of 0 is undefined. - In the third example, it illustrates the precision for very small values, highlighting the advantage of using
log1p()
.
- The first print statement outputs the logarithm of 1.0, which is
Practical Applications
Scientific Calculations
Describe the usage of
log1p()
in scenarios dealing with growth rates and other exponential calculations that require precise small value logging.Consider an example simulating an ecological growth model or financial interest computation.
javadouble growthFactor = 0.00001; // Small incremental growth double logGrowth = Math.log1p(growthFactor); System.out.println("Computed log growth factor: " + logGrowth);
This example effectively shows how
log1p()
can be used in scenarios where high precision is required for very small growth increments.
Error Log Computations
Discuss how to use
log1p()
for more accurate computation in error log handling in data processing or statistical computations.javadouble errorValue = 0.00003; // Example error value in computations double correctedLogError = Math.log1p(errorValue); System.out.println("Log corrected error: " + correctedLogError);
Here,
log1p()
provides precise results for logging very small errors, which can be critical in high-precision applications like statistical analysis and scientific research.
Conclusion
The log1p()
method in Java is an essential tool for situations requiring the calculation of logarithms where the argument is close to 0, ensuring higher precision and avoiding significant data loss. By incorporating log1p()
in suitable use cases—from scientific calculations involving small changes to error logging in sensitive data processes—you enhance the accuracy and reliability of your numerical computations. Apply the examples and concepts discussed to handle high-precision requirements efficiently in your Java applications.
No comments yet.