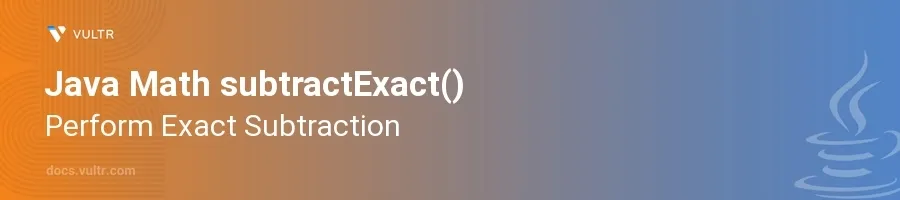
Introduction
Java's Math library includes various methods designed to handle arithmetic operations with precision and care for overflow, which can occur with large integer calculations. One such method is subtractExact()
, which performs subtraction, ensuring that the result is precisely what it mathematically should be, protecting against mathematical overflow by throwing exceptions when such cases occur.
In this article, you will learn how to use the subtractExact()
method in Java to handle subtraction operations accurately. This guide will help you understand how this method can be applied to your Java applications to ensure that calculations are safe from overflow issues in arithmetic operations.
Understanding the subtractExact() Method
Basic Usage
Import the Math class from the Java standard library.
Subtract two integers using the
subtractExact()
method and store the result.javaint result = Math.subtractExact(10, 5); System.out.println(result);
Here,
subtractExact(10, 5)
calculates the difference between 10 and 5, which is 5. This result is then printed.
Handling Overflow
Appreciate that integer overflow occurs when operations exceed the limits of the data type.
Use
subtractExact()
to catch potential overflows.javaint largeValue = Integer.MAX_VALUE; int result; try { result = Math.subtractExact(largeValue, -1); System.out.println(result); } catch (ArithmeticException e) { System.out.println("Overflow occurred: " + e.getMessage()); }
When trying to subtract -1 from
Integer.MAX_VALUE
, the operation tries to produce a result larger thanInteger.MAX_VALUE
, causing an overflow. This throws anArithmeticException
, which is caught and handled.
Using subtractExact() with Long Values
Acknowledge that
subtractExact()
also supports long values.Perform subtraction on long integers and observe behavior similar to integer handling.
javalong largeLongValue = Long.MAX_VALUE; long result; try { result = Math.subtractExact(largeLongValue, -1L); System.out.println(result); } catch (ArithmeticException e) { System.out.println("Overflow occurred: " + e.getMessage()); }
Similar to the integer example, this code attempts to subtract -1 from
Long.MAX_VALUE
, leading to an overflow for the 'long' data type and throwing anArithmeticException
.
Conclusion
Java's Math.subtractExact()
is an essential function for conducting accurate and safe arithmetic operations, particularly when working with large numbers where overflow is a concern. This method enhances the reliability and stability of an application by preventing subtle bugs related to arithmetic overflow. Utilizing subtractExact()
significantly aids in maintaining the integrity and correctness of mathematical calculations in Java programs. By mastering this tool, you ensure your application behaves predictably in situations where numerical limits might be challenged.
No comments yet.