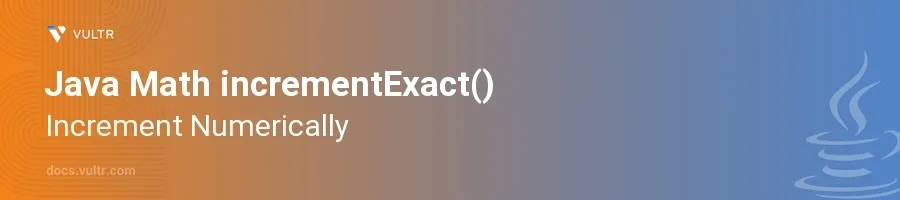
Introduction
The Java Math library provides various methods for performing arithmetic operations precisely, and incrementExact()
is one such method designed to increase a number by one. This method throws an exception if the resulting value overflows an int or long type, ensuring that developers handle unexpected behavior during arithmetic operations proactively.
In this article, you will learn how to use the incrementExact()
method effectively. Explore its application in various scenarios from simple counters to handling near-limit values in the range of integer or long primitives.
Using incrementExact() for Integer Values
Simple Increment
Start with a basic integer value.
Use
Math.incrementExact()
to increment the value by one.javaint value = 5; int incrementedValue = Math.incrementExact(value); System.out.println("Incremented Value: " + incrementedValue);
This code initializes
value
with a value of5
and then increments it to6
usingMath.incrementExact()
.
Boundary Condition with Integer
Initialize an integer to its maximum value.
Try incrementing it using
Math.incrementExact()
and catch the potentialArithmeticException
.javatry { int maxValue = Integer.MAX_VALUE; int result = Math.incrementExact(maxValue); System.out.println("Incremented Value: " + result); } catch (ArithmeticException e) { System.out.println("Cannot increment as it causes overflow: " + e.getMessage()); }
This example attempts to increment the maximum integer value. Since incrementing it would lead to overflow, it captures and handles the
ArithmeticException
.
Using incrementExact() for Long Values
Simple Increment on Long
Work with a long integer.
Apply
Math.incrementExact()
to increment the value.javalong longValue = 9999999999L; long incrementedLongValue = Math.incrementExact(longValue); System.out.println("Incremented Long Value: " + incrementedLongValue);
Here,
longValue
starts at9999999999
and increments by one without any overflow, as demonstrated by the outputs.
Boundary Condition with Long
Set a long to its maximum possible value.
Increment it while catching and handling the
ArithmeticException
.javatry { long maxLongValue = Long.MAX_VALUE; long result = Math.incrementExact(maxLongValue); System.out.println("Incremented Value: " + result); } catch (ArithmeticException e) { System.out.println("Cannot increment long as it causes overflow: " + e.getMessage()); }
Similar to the integer example, this tries to increment the maximum value of a
long
. TheArithmeticException
is caught, indicating an overflow error.
Conclusion
The Math.incrementExact()
method in Java ensures numerical precision by providing a safe way to increment integers and long values, with overflow detection. Utilizing this method helps prevent unnoticed errors in scenarios where precision in computation is critical. Implement Math.incrementExact()
in your numeric calculations to guarantee accurate results and robust applications, especially when working close to the limit values of numerical data types.
No comments yet.