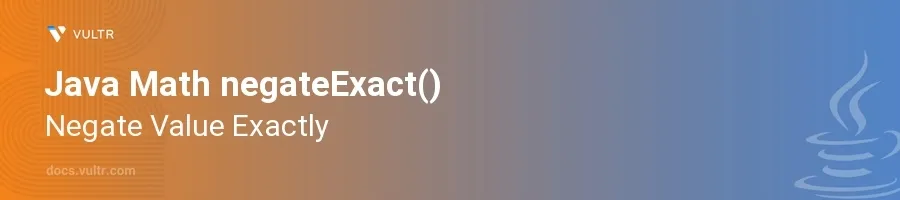
Introduction
In Java, the Math.negateExact()
method is a part of the Math class and provides an exact way to negate the value of integer and long types. This method is critical when you're dealing with calculations that require precise negation, especially in areas like financial calculations or systems programming where exactitude is paramount.
In this article, you will learn how to use the Math.negateExact()
method effectively in Java. You will explore how to handle basic negation tasks as well as manage situations where the negation of a value might lead to an overflow, thereby throwing an exception.
Basic Usage of negateExact()
Math.negateExact()
serves the simple purpose of returning the negation of the argument passed to it. If overflows occur (in scenarios with boundary values like Integer.MIN_VALUE
or Long.MIN_VALUE
), it throws an ArithmeticException
.
Negating an Integer
Pass an integer value to
Math.negateExact()
.Store or utilize the negated result.
javaint a = 10; int result = Math.negateExact(a); System.out.println("Negated Result: " + result);
This code will output
-10
since it negates the integer10
. The use ofnegateExact()
ensures that ifa
were at its boundary limits, any inappropriate calculation would raise an immediate flag via an exception.
Negating a Long Value
Handle a larger range by using a
long
type.Utilize
Math.negateExact()
for thelong
value similar to the integer example.javalong b = 200L; long result = Math.negateExact(b); System.out.println("Negated Result: " + result);
This will output
-200
. Just like with integers, negating a long ensures precision and error-checking for overflows.
Dealing with Overflow
Overflow can occur if you try to negate the minimum value for an integer or long since its positive counterpart (one unit larger in magnitude) cannot be represented in the same type.
Exception Handling in Negation
Prepare to handle the
ArithmeticException
caused by overflow.Use a try-catch block to capture and respond to the exception appropriately.
javatry { int result = Math.negateExact(Integer.MIN_VALUE); } catch (ArithmeticException e) { System.out.println("Exception: Cannot negate, overflow occurred."); }
In this example, negating
Integer.MIN_VALUE
would result in an overflow. TheArithmeticException
is caught and an appropriate message is displayed, preventing the program from crashing and allowing for a graceful error handling process.
Conclusion
The Math.negateExact()
method in Java is instrumental for precisely negating values while automatically managing potential overflows through exception handling. By utilizing this method, you ensure your mathematical operations are secure from overflow errors, which is especially beneficial in precision-critical applications. Use this method whenever you need an exact negation of integer or long values, and include exception handling strategies to guard against potential overflows. This approach maintains the robustness and reliability of your Java applications.
No comments yet.