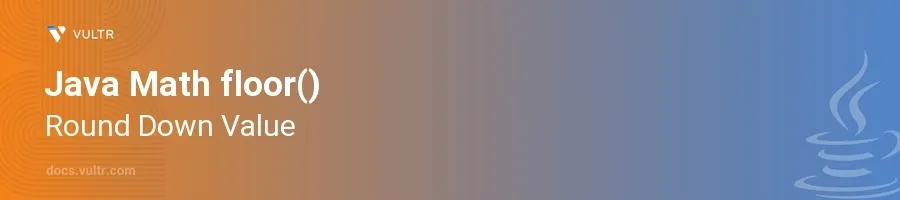
Introduction
The Math.floor()
method in Java is a crucial tool when it comes to rounding down floating-point numbers to the nearest integer that is less than or equal to the specified number. This function ensures that the result is the largest integer not greater than the argument and is widely used in scenarios where floor division or precise integer results are necessary from a double or float value.
In this article, you will learn how to utilize the Math.floor()
method in Java effectively. Explore various examples that demonstrate its functionality and understand the behavior of this method when applied to different types of numeric values.
Understanding Math.floor()
Basic Usage of Math.floor()
Start with a basic floating-point number.
Apply
Math.floor()
to round it down.javadouble result = Math.floor(8.76); System.out.println(result);
This code rounds down the number
8.76
to8.0
. Note that the result is still a double type.
Handling Negative Numbers
Use
Math.floor()
with a negative floating-point number.Observe how it rounds down to the nearest integer.
javadouble negativeResult = Math.floor(-2.95); System.out.println(negativeResult);
Here, the number
-2.95
is rounded down to-3.0
.Math.floor()
moves to the lower integer, which means a more negative number in this context.
Applying Math.floor() to Zero
Confirm the behavior of
Math.floor()
when applied to zero.Check the result.
javadouble zeroResult = Math.floor(0.0); System.out.println(zeroResult);
The output of
Math.floor(0.0)
is0.0
, demonstrating that zero remains unaltered, as it is already the lowest value it can round to.
Real-World Application Scenarios
Calculating Page Numbers for Pagination
Calculate how many pages are needed in a pagination system.
Use
Math.floor()
to ensure you get a whole number of pages.javadouble itemsPerPage = 10; double totalItems = 45; double totalPages = Math.floor(totalItems / itemsPerPage) + 1; System.out.println(totalPages);
With 45 items and 10 items per page, you'd calculate
4.5
pages. Rounding down gives4.0
and then add 1 to acknowledge partial pages, resulting in5.0
pages.
Image Processing
Calculate the dimensions needed to scale an image without changing its aspect ratio.
Use
Math.floor()
to avoid partial pixels.javadouble originalWidth = 1920; double scaleRatio = 0.75; double newWidth = Math.floor(originalWidth * scaleRatio); System.out.println(newWidth);
Scaling a 1920 pixel width image by 0.75 should result in 1440 pixels.
Math.floor()
ensures there are no fractional pixels in the width, which could affect image rendering.
Conclusion
The Math.floor()
function in Java is a powerful tool when accuracy and precision are needed in processing numerical data, ensuring results are consistently rounded down to the nearest integer. Whether handling simple numeric rounding or applying it to complex scenarios like pagination or image processing, Math.floor()
meets the demands of rigorous numeric operations without loss of integral data. By mastering its use, you maintain high levels of precision and predictability in your Java applications.
No comments yet.