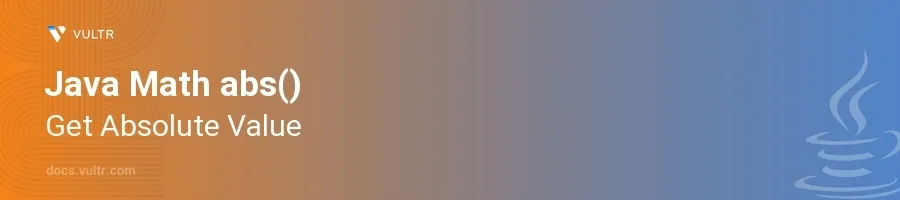
Introduction
The Math.abs()
method in Java is a widely used function that returns the absolute value of a given number, effectively removing any negative sign associated with it. This functionality is crucial in many applications, such as statistical computations, data analysis, and whenever it is necessary to ensure non-negative numerical values.
In this article, you will learn how to utilize the Math.abs()
method in Java. Discover how this method can be applied across various data types and see its importance in preventing errors in mathematical calculations that require positive numbers.
Understanding Math.abs()
Working with Integer Values
Determine the absolute value of an integer. Negative signs are removed, and positive integers remain unchanged.
Invoke the
Math.abs()
method with an integer as an argument.javaint result = Math.abs(-10); System.out.println(result);
This code outputs
10
, demonstrating howMath.abs()
converts a negative integer to its positive counterpart.
Handling Floating Point Numbers
Use
Math.abs()
to process floating-point numbers, such asfloat
anddouble
.Apply the method to a double or float to get the absolute value.
javadouble result = Math.abs(-123.45); System.out.println(result);
Here, the output is
123.45
, showing how the method handles floating point numbers by stripping away the negative sign.
Applying Math.abs() in Real-World Scenarios
Utilize the
Math.abs()
method in practical situations like error margins or distances where only positive values make sense.Compute scenarios where the direction (negative or positive) does not matter but the magnitude does.
- Comparing prices' differences regardless of order
- Determining whether a point lies within a certain radius of a central point
- Calculating the time elapsed (always positive) between events
Edge Cases and Considerations
Understanding Limitations and Behavioral Quirks
Beware of the edge cases like
Integer.MIN_VALUE
orDouble.NaN
.Recognize that in Java, the absolute value of
Integer.MIN_VALUE
(-2147483648
) remains negative due to overflow.javaint minValueAbs = Math.abs(Integer.MIN_VALUE); System.out.println(minValueAbs);
Here, the result is still
-2147483648
, an important exception to be aware of since it does not behave as one might intuitively expect.
Dealing with NaN and Infinite Values
Acknowledge that when applied to
NaN
or infinite values in floating point calculations,Math.abs()
will returnNaN
or the positive infinity, respectively.javadouble nanValueAbs = Math.abs(Double.NaN); System.out.println(nanValueAbs); double infinityValueAbs = Math.abs(Double.NEGATIVE_INFINITY); System.out.println(infinityValueAbs);
This demonstrates that the method correctly handles special floating-point numbers, maintaining logical consistency in mathematical operations.
Conclusion
The Math.abs()
function in Java is invaluable for ensuring that numerical values remain positive or for removing the directionality from measurements and calculations. It provides a straightforward way to deal with absolute values for integers, floats, and doubles, supporting various mathematical and real-world applications. Understanding its behavior across different data types and recognizing potential edge cases ensures effective and error-free numerical operations in your Java programs.
No comments yet.