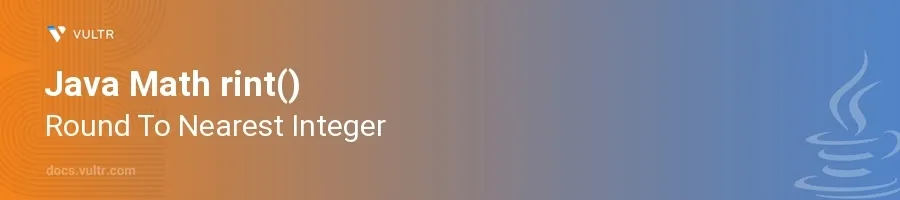
Introduction
The rint()
method in Java is a built-in function in the Math
class that is used to round a double value to the nearest integer using conventional rounding rules. This function adheres to half-up rounding, where values that are exactly midway between two integers are rounded towards the nearest even integer. This type of rounding is also known as "rounding half even" and is particularly useful in reducing cumulative rounding error in large sequences of calculations.
In this article, you will learn how to effectively utilize the rint()
function in Java. Explore how this function operates with different double values, and understand its behavior during the rounding process of positive, negative, and boundary values.
Round Various Types of Float Values
Round Positive Floating-Point Numbers
Define positive floating-point numbers.
Apply the
rint()
method.javadouble posFloat = 8.5; double roundedPos = Math.rint(posFloat); System.out.println(roundedPos);
This code rounds the floating-point number
8.5
to8.0
, demonstrating the rounding half even behavior where.5
rounds to the nearest even number.
Round Negative Floating-Point Numbers
Consider negative values to see the effect of
rint()
.Use the method on these values.
javadouble negFloat = -2.5; double roundedNeg = Math.rint(negFloat); System.out.println(roundedNeg);
Here, the floating-point number
-2.5
is rounded to-2.0
. This example shows thatrint()
handles negative numbers by rounding half to the nearest even number, similar to positive numbers.
Handle Boundary Cases
Test values that stand right on the boundary of rounding rules.
Observe how
rint()
resolves these cases.javadouble boundaryFloat = 2.5001; double roundedBoundary = Math.rint(boundaryFloat); System.out.println(roundedBoundary);
For the value
2.5001
, the function rounds up to3.0
since it's slightly above the midpoint.
Using rint() in Financial Calculations
Avoid Cumulative Rounding Errors
Simulate a scenario where numerous roundings may lead to a significant error.
Apply
rint()
to maintain accuracy across multiple operations.javadouble financialCalc = 0.1 + 0.1 + 0.1; // typically might not sum to exactly 0.3 double exactFinancialCalc = Math.rint(financialCalc * 10) / 10; System.out.println(exactFinancialCalc);
In this example, small imprecisions inherent in floating-point arithmetic can lead to errors. By using
rint()
, you manage to keep these errors minimal, demonstrating the method’s utility in scenarios requiring high precision like financial transactions.
Conclusion
The rint()
function in Java is crucial for rounding double values to the nearest integer according to the half-even rule. It ensures precision, especially in scenarios with potential cumulative errors, such as in financial calculations. By following the explained examples and techniques, optimize rounding processes in your applications to achieve more reliable and accurate results. Whether it's handling edge cases or everyday numeric data, Math.rint()
stands as a robust option.
No comments yet.