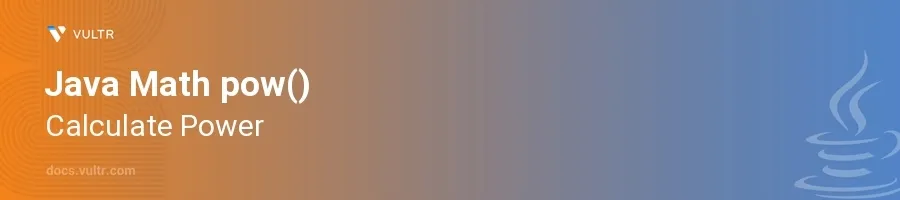
Introduction
The Math.pow()
method in Java is a powerful utility for performing exponentiation, that is, raising a base number to the power of an exponent. This function is part of the java.lang.Math
class, which provides a wide range of mathematical operations and constants. Math.pow()
is commonly used in scientific calculations, financial computations, and anywhere else precise exponentiation is required.
In this article, you will learn how to use the Math.pow()
method in various scenarios. Explore its basic usage, understand its behavior with different data types, and see how it interacts with negative and fractional exponents to efficiently solve complex mathematical problems in Java.
Basic Usage of Math.pow()
Calculating a Simple Power
- Define the base and the exponent.
- Call
Math.pow(base, exponent)
to compute the power. - Print the result to observe the output.
double base = 2;
double exponent = 3;
double result = Math.pow(base, exponent);
System.out.println("2 raised to the power of 3 is: " + result);
This code snippet computes (2^3), resulting in 8.0. Here, Math.pow()
takes two double arguments and returns the base raised to the power of the exponent as a double.
Handling Different Data Types
- Use integer values that Java implicitly converts to double.
- Apply
Math.pow()
with these integer values.
int intBase = 5;
int intExponent = 2;
double result = Math.pow(intBase, intExponent);
System.out.println("5 raised to the power of 2 is: " + result);
In this example, even though Math.pow()
expects double arguments, Java's automatic type conversion allows integers to be used directly. Math.pow(5, 2)
effectively computes (5^2), returning 25.0.
Fractional and Negative Exponents
- Calculate roots using fractional exponents.
- Demonstrate the effect of negative exponents.
double base = 9;
double rootExponent = 0.5; // equivalent to square root
double negativeExponent = -2; // equivalent to reciprocal of the square
double rootResult = Math.pow(base, rootExponent);
double reciprocalResult = Math.pow(base, negativeExponent);
System.out.println("Square root of 9 is: " + rootResult);
System.out.println("Reciprocal of 9 squared is: " + reciprocalResult);
This code first calculates the square root of 9 using an exponent of 0.5, resulting in 3.0. It then calculates the reciprocal of 9 squared by using an exponent of -2, which yields approximately 0.012345679 (or (1/81)).
Advanced Applications of Math.pow()
Exponential Growth Calculation
- Simulate exponential growth scenarios, such as compound interest.
- Set up the variables for the base growth rate and the time period.
- Calculate the growth using
Math.pow()
.
double principal = 1000; // Initial amount
double growthRate = 1.05; // 5% growth per period
int periods = 10; // Number of periods
double futureValue = principal * Math.pow(growthRate, periods);
System.out.println("Future value after 10 periods: " + futureValue);
This example calculates the future value of an initial investment of $1000 growing at 5% per period over 10 periods. The use of Math.pow()
simplifies the calculation of compounding growth rates.
Conclusion
The Math.pow()
method in Java is a versatile function for performing exponentiation. Its ability to handle different types of inputs and calculations makes it essential in many programming scenarios, from performing simple arithmetic to solving complex mathematical problems. By mastering Math.pow()
, you enhance your Java programming skills and can handle a variety of mathematical tasks more efficiently. Consider the insights and examples discussed to implement robust solutions in your Java applications.
No comments yet.