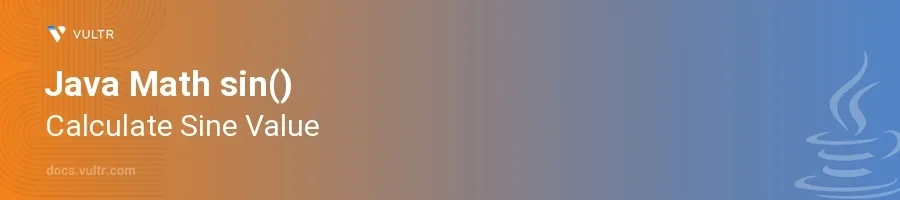
Introduction
The Math.sin()
method in Java is an essential function that calculates the sine of a specified angle. This function takes an angle in radians and returns the sine value, which is crucial in various fields ranging from engineering to computer graphics where trigonometric functions play a pivotal role.
In this article, you will learn how to use the Math.sin()
method to calculate the sine values of angles. Through a series of examples, you’ll understand how to implement this function in both simple and complex contexts and explore the precision of the output angles.
Calculating Sine Values in Java
Basic Sine Value Calculation
Convert an angle from degrees to radians using
Math.toRadians()
.Apply the
Math.sin()
method to compute the sine value.javadouble degrees = 90; double radians = Math.toRadians(degrees); double sineValue = Math.sin(radians); System.out.println("Sine of " + degrees + " degrees is: " + sineValue);
This code snippet converts 90 degrees to radians and calculates the sine value. The
Math.sin()
method requires the angle in radians, thus necessitating the conversion.
Sine Values for Variable Angles
Use a loop to calculate sine values of multiple angles.
javafor (int i = 0; i <= 360; i += 90) { double radians = Math.toRadians(i); double sineValue = Math.sin(radians); System.out.println("Sine of " + i + " degrees is: " + sineValue); }
This example calculates and prints the sine values for angles 0, 90, 180, 270, and 360 degrees, demonstrating how
Math.sin()
handles various common angles in trigonometry.
Handling Negative Angles and Full Circle Calculations
Convert negative angles and angles greater than 360 degrees to their proper equivalents within the 0 to 360 degrees range.
javadouble[] testAngles = {-45, 360, 450}; for (double angle : testAngles) { double normalizedAngle = angle % 360; double radians = Math.toRadians(normalizedAngle); double sineValue = Math.sin(radians); System.out.println("Sine of " + angle + " degrees (normalized to " + normalizedAngle + " degrees) is: " + sineValue); }
This snippet normalizes the angles -45 degrees, 360 degrees, and 450 degrees to their equivalents within the standard circle (0-360 degrees) and computes their sine values accordingly.
Conclusion
The Math.sin()
function in Java is a powerful tool for trigonometric calculations. It's essential to remember that this method requires input in radians, which you can obtain by converting degrees using Math.toRadians()
. With the ability to handle any angle, whether negative or exceeding 360 degrees, Math.sin()
ensures that your calculations remain accurate and straightforward. Utilize this function whenever you need to determine sine values in your applications, ensuring that trigonometric computations are both precise and efficient.
No comments yet.