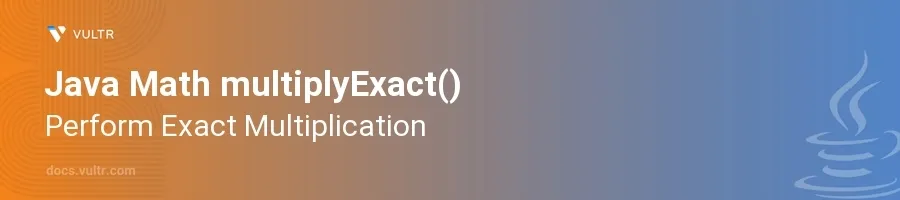
Introduction
The multiplyExact()
method in Java is part of the Math class, providing an essential utility to perform multiplication that exactly results in an integer or long value. This method throws an exception if the product overflows the int or long type bounds, thus ensuring safe computations where exact results are crucial, such as financial calculations or system control applications.
In this article, you will learn how to effectively use the multiplyExact()
method across different programming scenarios. Discover ways this method can prevent errors in operations that may exceed the maximum values storable in integer types and understand the repercussions of such overflows.
Understanding multiplyExact() Usage
Basic Multiplication
Use
multiplyExact()
with integer values to perform safe multiplication.Test the multiplication for potential overflows.
javaint a = 15000; int b = 2000; int result = Math.multiplyExact(a, b); System.out.println("The product is: " + result);
This code multiplies two integer values. If the result overflows the
int
bounds, anArithmeticException
is thrown.
Error Handling with multiplyExact()
Prepare to handle possible overflows using try-catch blocks.
Employ the method within a
try
block and catch any resultingArithmeticException
.javatry { int a = Integer.MAX_VALUE; int b = 2; int result = Math.multiplyExact(a, b); System.out.println("The product is: " + result); } catch (ArithmeticException e) { System.err.println("Error: " + e.getMessage()); }
This example attempts to multiply
a
andb
, which would result in an overflow of the maximum integer size. The catch block catches and handles theArithmeticException
, allowing the program to continue gracefully.
Using multiplyExact() with Long Values
Utilize
multiplyExact()
for multiplication with long values.Handle overflows in a similar fashion as integer overflows.
javalong x = 922337203685477580L; long y = 10L; long result = Math.multiplyExact(x, y); System.out.println("The product is: " + result);
Over here,
multiplyExact()
checks for overflow in long multiplication which is similarly handled by throwing anArithmeticException
if an overflow occurs.
Application Scenarios
Financial Calculations
Ensure precise computation in scenarios like monetary transactions or budget analyses.
Enhance the reliability of financial applications by preventing integer overflows.
javaint pricePerItem = 299; int quantity = 1000000; int totalCost = Math.multiplyExact(pricePerItem, quantity); System.out.println("Total cost is: " + totalCost);
This code safely computes a potentially large multiplication involving quantities and costs, which is common in financial applications.
Robust System Controls
Apply
multiplyExact()
to manage system resources or control parameters where exact value computation is required.Avoid system malfunctions or erroneous behavior caused by value overflows.
javaint controlFactor = 123456; int scaleFactor = 1000; int adjustedControl = Math.multiplyExact(controlFactor, scaleFactor); System.out.println("Adjusted Control Value: " + adjustedControl);
In control systems, precise computation is necessary to maintain system integrity and functionality.
multiplyExact()
aids in achieving these precise calculations without risking overflows.
Conclusion
Utilizing the multiplyExact()
method in Java ensures that your computations remain within the safe bounds of integer and long arithmetic, crucially reducing the risk of overflow errors in your applications. Whether applied in financial computations or critical system controls, multiplyExact()
provides a reliable way to handle large multiplications safely. By implementing the approaches discussed, you achieve robustness and reliability in your Java applications, ensuring they perform accurately and predictably under various circumstances.
No comments yet.