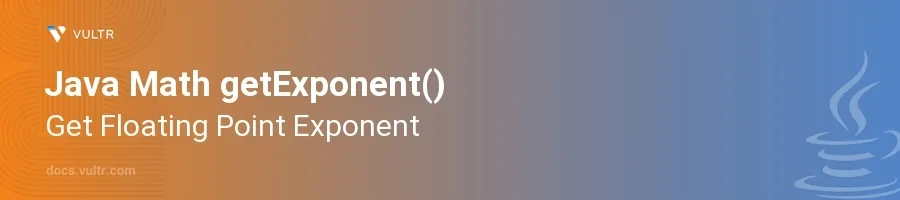
Introduction
In Java, the Math.getExponent()
method is part of the Math class and provides a straightforward means to retrieve the unbiased exponent used in the representation of a floating point number, according to the IEEE 754 floating-point "double format" bit layout. This method is particularly useful in scenarios where you need to analyze or manipulate the components of a floating-point number at a low level.
In this article, you will learn how to use the Math.getExponent()
method in Java. Explore how to apply this method with both double and float types, and understand its behavior through practical examples.
Utilizing Math.getExponent() with Double
Extract the Exponent from a Double Value
Declare and initialize a double variable.
Use
Math.getExponent()
to find the exponent part of the double value.javadouble value = 150.45; int exponent = Math.getExponent(value); System.out.println("Exponent of " + value + " is: " + exponent);
This code snippet prints the exponent of the
value
. The output will show the exponent based on the representation of the double in binary format.
Understanding the Output
- Understand that the exponent derived is in line with the IEEE 754 standard.
- Realize that
Math.getExponent()
returns the exponent part used in the binary representation of the floating-point number, which corresponds to the formula (2^{exponent}) parts of the number.
Working with Math.getExponent() on Float Types
Extract the Exponent from a Float Value
Initialize a float variable.
Apply
Math.getExponent()
and print the result.javafloat floatValue = 32.75f; int floatExponent = Math.getExponent(floatValue); System.out.println("Exponent of " + floatValue + " is: " + floatExponent);
Here, the exponent part of
floatValue
is calculated and displayed. It works similarly to double values but uses the floating-point representation for float.
Interpretation of Results
- Acknowledge that the output follows the same IEEE 754 standard but for float.
- The exponent output represents the binary representation where the float is broken down into its scientific notation equivalent.
Use Cases of getExponent()
Analyzing Scientific Data
- Use
getExponent()
when accurate scientific computation necessitates manipulation of exponent parts directly. - Helpful in fields like astronomy and physics where precision and exponentiation are common tasks.
Debugging Floating-Point Operations
- Use this method to debug and verify the correctness of floating-point operations.
- Especially useful when operations could lead to loss of precision or floating-point errors.
Optimization and Performance Checks
- Determine specific exponent ranges that might need special handling in optimizing algorithms.
- Useful in algorithms that scale differently based on the magnitude of input numbers.
Conclusion
The Math.getExponent()
function in Java is a powerful utility to extract the exponent part of floating-point numbers directly. It adheres to the IEEE 754 standard, providing consistency across different computing platforms. By incorporating this function, you enhance your capability to manipulate and analyze floating-point numbers in scientific computing, debugging, and performance optimization scenarios. Use the techniques discussed to effectively handle precise computation tasks and optimize floating-point operations in Java.
No comments yet.