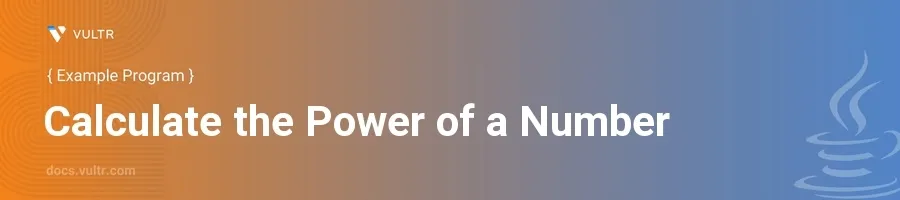
Introduction
Calculating the power of a number is a common task in programming and mathematics, involving raising a base number to the power of an exponent. In Java, this operation can be handled in multiple ways, depending on the precision and size of the numbers involved. This article explores how to implement power calculations in Java using different methods, each suitable for various scenarios.
In this article, you will learn how to implement power calculations in Java through examples. You'll explore different methods such as using a loop, the Math.pow()
function, and recursion. These examples will provide a clear understanding of the techniques and their appropriate use cases.
Using Java's Math.pow() Method
Calculate the Power Using Math.pow()
Import the
Math
package.Define the base and the exponent.
Use
Math.pow()
to calculate the power.javadouble base = 2.0; int exponent = 5; double result = Math.pow(base, exponent); System.out.println("Result: " + result);
This code snippet uses the
Math.pow()
method to calculate 2 raised to the power of 5, which results in 32.0. The method is highly efficient and handles both positive and negative exponents.
Using Loops to Calculate Power
Implement Power Calculation with a For Loop
Define base and exponent variables.
Initialize a result variable to 1.
Use a for loop to multiply the result by the base for the number of times specified by the exponent.
javaint base = 3; int exponent = 4; int result = 1; for (int i = 0; i < exponent; i++) { result *= base; } System.out.println("Result: " + result);
In this example, the loop iterates four times, multiplying the result by 3 each time, leading to a final result of 81.
Using Recursion to Calculate Power
Recursive Method for Power Calculation
Create a method
power
that takes two arguments: base and exponent.Add base conditions to handle cases where the exponent is 0 or 1.
Use recursion to reduce the exponent in each call.
javapublic class PowerCalculator { public static int power(int base, int exponent) { if (exponent == 0) return 1; // Any number to the power of 0 is 1 if (exponent == 1) return base; // Base case for recursion return base * power(base, exponent - 1); } public static void main(String[] args) { int base = 2; int exponent = 8; int result = power(base, exponent); System.out.println("Result: " + result); } }
This example defines a recursive method
power
that continues to call itself with a reduced exponent until it reaches a base condition. The power of 2 raised to 8 is calculated in this recursive manner, resulting in 256.
Conclusion
Calculating the power of a number can be approached in various ways in Java, depending on the specific requirements and constraints of your project. Methods like using Java's built-in Math.pow()
function, implementing a loop, or using a recursive function each have their advantages. Familiarize yourself with these techniques to select the most appropriate method for your situation, ensuring your Java programs are efficient and capable of handling power calculations effectively.
No comments yet.