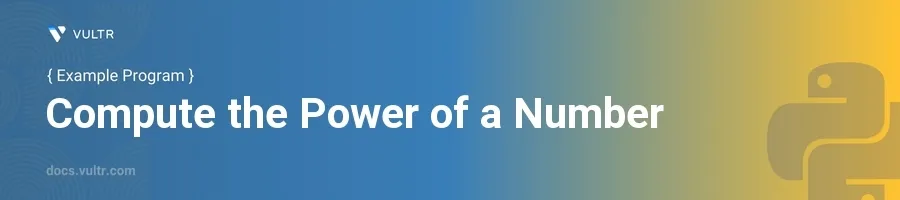
Introduction
Calculating the power of a number is a fundamental operation in mathematics and many scientific applications. In programming, this operation can be performed easily using Python, which supports various methods to raise a number to a power. This is commonly needed in areas such as physics calculations, algorithm complexity estimations, and financial projections.
In this article, you will learn how to compute the power of a number using Python. Explore different approaches, including the use of built-in functions and logical constructs, to handle this mathematical operation effectively.
Using the Built-in pow
Function
Compute Power with Two Arguments
Recognize that Python's built-in
pow()
function can compute power in the form ofpow(base, exp)
wherebase
is the number andexp
is the exponent.Call the
pow()
function with the base and the exponent.pythonbase = 2 exponent = 5 result = pow(base, exponent) print(result)
The
pow(base, exponent)
function calculates (2^5), which equals 32. This method directly uses Python's built-in capability to compute powers.
Using Three Arguments for Modular Exponentiation
Understand that
pow()
can also handle three arguments,pow(base, exp, mod)
, which returns(base ** exp) % mod
.Utilize the three-argument version to calculate the power, then apply the modulus operation.
pythonbase = 3 exponent = 4 modulus = 5 result = pow(base, exponent, modulus) print(result)
Here, (3^4 % 5) is computed, which results in (81 % 5) equal to 1. This approach is particularly useful in cryptographic computations where modular exponentiation is common.
Using Arithmetic Operators
Simple Power Computation
Use the
**
operator for exponentiation, which is straightforward and commonly used in Python.Apply the operator to calculate the power of a number.
pythonbase = 5 exponent = 3 result = base ** exponent print(result)
This code computes (5^3), which equals 125. The
**
operator is intuitive and efficient for simple exponentiations.
Handling Negative and Fractional Exponents
Compute Negative Exponents
Be aware that Python handles negative exponents, which correspond to calculating the reciprocal of the base raised to the positive exponent.
Write the calculation for a negative exponent.
pythonbase = 4 exponent = -2 result = base ** exponent print(result)
The calculation (4^{-2}) yields ( \frac{1}{16} ) or 0.0625. It effectively computes the inverse of the square of the base.
Fractional Exponents to Compute Roots
Realize that fractional exponents can calculate roots, such as square roots, cube roots, etc.
Set up a calculation for a fractional exponent.
pythonbase = 16 exponent = 0.5 result = base ** exponent print(result)
This code computes (16^{0.5}), which is the square root of 16, yielding a result of 4.
Conclusion
Computing the power of a number in Python is a straightforward process whether using the built-in pow
function or the arithmetic **
operator. The flexibility of these methods allows you to handle positive, negative, and fractional exponents effectively. Utilize these techniques in various applications ranging from simple mathematical calculations to complex scientific computing. By mastering these methods, ensure your computations are efficient and accurate within your Python projects.
No comments yet.