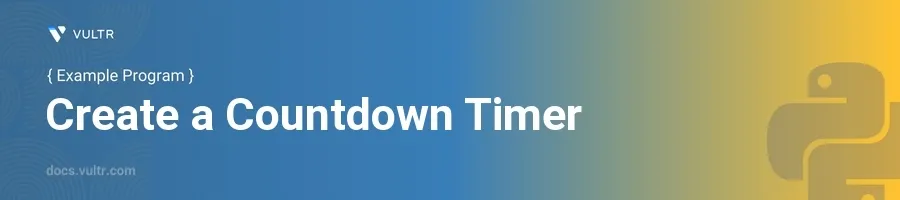
Introduction
A countdown timer is a sequence that counts in reverse from a specified time allocation to zero, and it can be implemented in several different programming environments, with Python being particularly straightforward thanks to its rich standard libraries. Programmatically, a countdown timer can be extremely useful in applications such as time management tools, game development, and during timed tests or sessions.
In this article, you will learn how to create a basic countdown timer using Python. Discover methods to implement this functionality with clear examples to ensure you can integrate a timer into your Python projects efficiently.
Building a Simple Countdown Timer
Set Up the Timer Function
Import Python's
time
module, which is necessary for the timer’s sleep functionality.Define a function to encapsulate the countdown logic. This function will take one argument: the number of seconds to count down from.
pythonimport time def countdown(t): while t: mins, secs = divmod(t, 60) timer = '{:02d}:{:02d}'.format(mins, secs) print(timer, end="\r") time.sleep(1) t -= 1 print("Time's up!")
This function counts down in seconds and formats the output as minutes and seconds. The
print
function uses\r
to ensure each new timer value overwrites the previous one, keeping the console output neat.
Using the Countdown Function
Call the countdown function by passing the desired time in seconds.
pythoncountdown(90)
This call sets the timer for 1 minute and 30 seconds. When executed, it will display a countdown in your console.
Enhancing the Timer with User Input
Extend the Timer to Accept User Input
Enhance the timer by allowing the user to input the time in seconds or minutes for flexibility.
pythondef user_countdown(): t = input("Enter the time in seconds: ") countdown(int(t))
By adding a simple input function, users can now define how long the timer should run.
Implementing and Testing User Interaction
Now, execute the user-driven countdown timer function to see it in action.
pythonuser_countdown()
Running this function will prompt the user to enter a countdown duration, making the timer more interactive and versatile.
Conclusion
Implementing a countdown timer in Python is not only straightforward but also an excellent practice for understanding basic programming concepts such as loops, time management, and user interaction. With the examples provided, equip your applications with time-sensitive features to enhance user experience or to enforce time-based rules. Explore further by integrating this timer into larger projects or by expanding its capabilities with additional features like audible alarms or graphical user interfaces.
No comments yet.