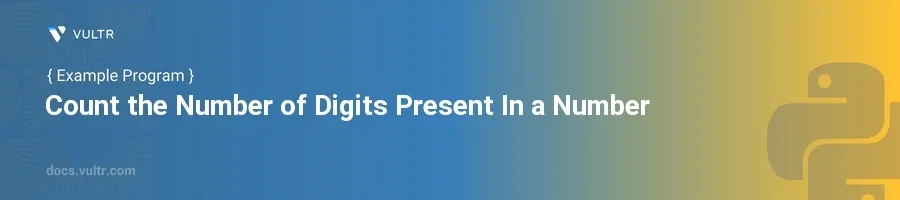
Introduction
Counting the number of digits in a number is a fundamental operation in many programming scenarios, such as validating input, processing numerical data, or implementing algorithms that depend on the length of numbers. This operation can be performed in various ways using Python, demonstrating the language's flexibility and power in handling numbers and strings.
In this article, you will learn how to write a Python program to count the number of digits in a number using different methods. Explored techniques include converting numbers to strings, utilizing mathematical operations, and leveraging Python's built-in functions.
Python Program to Count the Total Number of Digits in a Number Using For Loop
Here's a Python program to count the total number of digits in a number using a for loop:
The program takes an integer input from the user.
It converts the number to a string (handling negative numbers with abs()).
A for loop iterates over each digit, increasing the count.
Finally, it prints the total number of digits.
pythonnum = int(input("Enter a number: ")) count = 0 for digit in str(abs(num)): count += 1 print("Total number of digits:", count)
Count No of Digits in Python by Converting to String
Example: Using String Length
Convert the number to a string.
Calculate the length of the string.
pythonnumber = 12345 number_str = str(number) digit_count = len(number_str) print(digit_count)
By converting the number to a string, you enable the use of the
len()
function, which returns the number of characters in the string, effectively counting the digits.
Counting Digits Using Mathematical Logic
Example: Using While Loop and Division
Initialize a count variable to zero.
Use a while loop that executes as long as the number is greater than zero.
Inside the loop, divide the number by 10 and increment the count by one.
pythonnumber = 12345 count = 0 while number > 0: number //= 10 count += 1 print(count)
In this method, each division by 10 removes one digit from the number, and the count variable keeps track of how many digits have been processed.
Program to Count the Number of Digits in Python Using Functions
Example: Using math.log()
Import the
math
module.Calculate the logarithm base 10 of the number.
Take the floor of the logarithm result and add one to get the number of digits.
pythonimport math number = 12345 if number > 0: digit_count = math.floor(math.log10(number)) + 1 elif number == 0: digit_count = 1 else: digit_count = math.floor(math.log10(-number)) + 1 # Handle negative numbers print(digit_count)
This method leverages logarithmic properties to calculate digit length mathematically. Logarithm base 10 of a number gives the number of times you can divide that number by 10 before you get a result less than 1, which correlates to the number of digits.
Conclusion
Counting the number of digits in a number can be accomplished through various approaches in Python, each suitable for different contexts and preferences. Whether transforming the number into a string, using straightforward iterative division, or applying a mathematical function, each method effectively achieves the desired outcome. Choose the approach that best suits your specific requirements for readability, performance, and ease of implementation to maintain clean, effective code.
No comments yet.