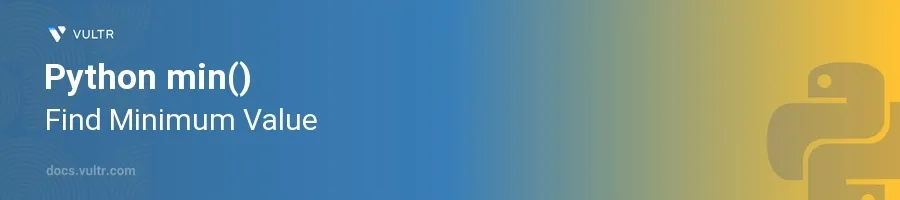
Introduction
The min()
function in Python is a straightforward yet powerful tool used to determine the smallest item in an iterable or the smallest of two or more arguments. This function plays a crucial role in various areas such as data analysis, algorithm development, and everyday programming tasks where finding the minimum value is necessary.
In this article, you will learn how to effectively utilize the min()
function across different scenarios. Delve into the basics of this function, see it applied to sequences like lists and tuples, and discover how to customize its behavior with key functions for more complex data types.
Using min() on Lists and Tuples
Find the Minimum in a List
Create a list of numerical values.
Use the
min()
function to find the smallest number in the list.pythonnumbers = [5, 10, 1, 7, 4] smallest_number = min(numbers) print(smallest_number)
This code initializes a list named
numbers
and finds the smallest value usingmin()
. The result is1
, as it is the least number in the list.
Find the Minimum in a Tuple
Define a tuple with several elements.
Use the
min()
function to determine the smallest element.pythondata = (8, 3, 2, 50, 12) min_element = min(data) print(min_element)
In this scenario,
min()
evaluates the elements of the tupledata
and returns2
, the smallest element.
Using min() with Strings
Find the Lexicographically Smallest String
Define a list of string elements.
Apply the
min()
function to obtain the lexicographically smallest string.pythonnames = ["Alice", "Bob", "Diana", "Eli"] smallest_name = min(names) print(smallest_name)
Here,
min()
examines the strings in the listnames
. "Alice" is returned as it comes first lexicographically among the names.
Customizing min() with Key Functions
Using a Key Function for Complex Data Types
Define a list of dictionaries representing animals and their weights.
Use the
min()
function with a key function to find the animal with the least weight.pythonanimals = [ {'name': 'Dog', 'weight': 40}, {'name': 'Cat', 'weight': 5}, {'name': 'Elephant', 'weight': 1000} ] lightest_animal = min(animals, key=lambda x: x['weight']) print(lightest_animal)
This snippet uses a lambda function as a key to specify that the comparison should be done based on the 'weight' of each animal. The result shows that the cat is the lightest animal with a weight of 5.
Conclusion
The min()
function in Python is an essential tool for efficiently finding the minimum values in various iterable structures and more complex scenarios. By mastering this function, you enhance your ability to write cleaner and more efficient Python code. Whether you're dealing with simple lists or more structured data like dictionaries, min()
ensures that you can easily extract the smallest element tailored to your specific needs. Implement these methods in your projects to manage and analyze data more effectively.
No comments yet.