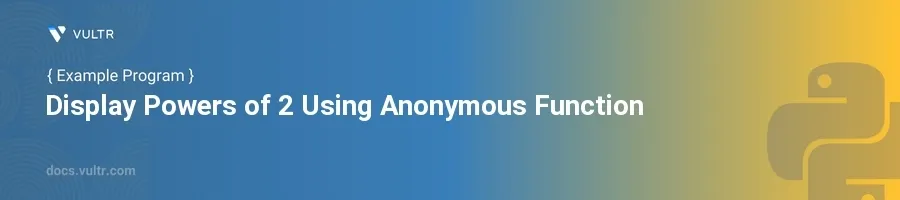
Introduction
Anonymous functions in Python, often defined using the lambda
keyword, are compact, inline functions that contain a single expression. They are particularly useful for short, quick function definitions typically used where functions are needed temporarily. This feature is especially handy when working with mathematical operations like calculating powers.
In this article, you will learn how to use Python's anonymous functions to display powers of 2. Through practical examples, understand how to efficiently generate and print the powers of 2 using simple and concise code.
Displaying Powers of 2
Basic Usage of Lambda Functions
Anonymous functions are defined using the lambda
syntax. To demonstrate their utility in calculating powers, start with a basic example calculating a single power of 2.
Define a lambda function that takes an exponent and returns 2 raised to the power of that exponent.
Print the result for a given power.
pythonpower_of_2 = lambda x: 2 ** x print(power_of_2(3)) # Outputs 8
In this example,
power_of_2
computes 2 raised to the power of 3, returning 8.
Using a List Comprehension with Lambda
To display a series of powers of 2, combine lambda functions with list comprehensions. This approach is ideal for generating a list of results over an iterable.
Create a lambda function as before.
Use a list comprehension to apply this function over a range of values.
Print the resulting list of powers of 2.
pythonpowers_of_2_list = [lambda x: 2 ** x for x in range(10)] results = [func(0) for func in powers_of_2_list] print(results) # Outputs the first 10 powers of 2
Each element of
powers_of_2_list
is a lambda function set to calculate 2 raised to a power, whererange(10)
defines the powers from 0 to 9. The results are collected and printed, showing all calculated powers.
Replicating a Function with Map
The map()
function can be used in Python to apply a function to every item in an iterable. Leverage this with lambda for an elegant solution.
Define the lambda function to compute the power of 2.
Use
map()
to apply this lambda function across a desired range of exponent values.Convert the map object to a list and print it.
pythonexponents = range(10) powers = map(lambda x: 2 ** x, exponents) print(list(powers))
Here,
map()
takes two arguments: a function and an iterable. The anonymous function is applied to each item inexponents
, effectively calculating the powers of 2 from 0 to 9.
Conclusion
Anonymous functions in Python provide a streamlined, efficient mechanism for performing operations like calculating powers of 2, especially useful in scenarios involving iteration and application of a single function to many inputs. By following the examples provided here, you can effectively utilize lambda functions combined with list comprehensions or the map function to simplify your Python scripts, maintaining clear and concise code. Whether for a small script or part of a larger project, these techniques help keep your codebases maintainable and readable.
No comments yet.