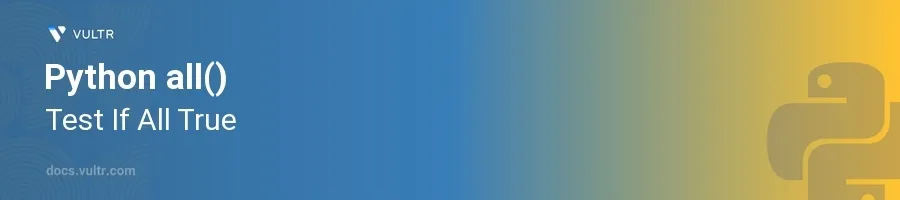
Introduction
The all()
function in Python is critical for determining whether every element within an iterable (like lists, tuples, or dictionaries) meets a condition, specifically that all elements are True
. This function is highly advantageous in cases where a collective status needs affirmation, such as validating configurations, ensuring completeness of data, or applying conditions across multiple inputs.
In this article, you will learn how to efficiently use the all()
function in various programming scenarios. Explore this function's application with different data types and structures such as lists, tuples, and dictionaries, understanding how it enhances code readability and reliability.
Using all() with Lists
Test Every Element for Truth Value
Create a list of boolean values.
Use the
all()
function to evaluate if all items in the list areTrue
.pythonbool_list = [True, True, True, True] result = all(bool_list) print(result)
This snippet tests the list
bool_list
to check if every item isTrue
.all()
returnsTrue
since all values meet the condition.
Combining Conditions in Lists
Formulate a list where each element is a condition.
Apply the
all()
function to determine if all conditions are true.pythonx = 5 y = 10 conditions = [x < 10, y > 5, x + y == 15] result = all(conditions) print(result)
In this example, all conditions in the list
conditions
areTrue
(x
is less than 10,y
is greater than 5, and their sum is 15), henceall()
yieldsTrue
.
Using all() with Tuples
Ensure All Tuple Elements are Non-Zero
Understand that
all()
views any non-zero value asTrue
.Create a tuple of numeric values where none are zero.
Use
all()
to test these values.pythonnum_tuple = (1, 2, 3, 4) result = all(num_tuple) print(result)
Here, since no elements in
num_tuple
are zero,all()
returnsTrue
, proving all values are effectivelyTrue
(non-zero).
Using all() with Dictionaries
Validate All Dictionary Values
Acknowledge that
all()
by default examines only the keys in a dictionary, but can be used with values by invokingdict.values()
.Construct a dictionary and check all values with specific criteria.
Use
all()
in conjunction withdict.values()
.pythondict_data = {'a': 10, 'b': 20, 'c': 30} result = all(value > 5 for value in dict_data.values()) print(result)
In this dictionary
dict_data
, all values exceed 5, so usingall()
on the generator expression that checks each value assures that every condition passes, thus returningTrue
.
Conclusion
The all()
function is a versatile utility in Python, indispensable for validating that each element in an iterable satisfies a specified condition. Whether checking configurations, verifying multiple conditions, or ensuring that all elements in a collection are aligned with a requirement, all()
serves to streamline complex checks into concise and manageable code segments. By integrating the strategies discussed, you enhance your program's robustness and clarity.
No comments yet.