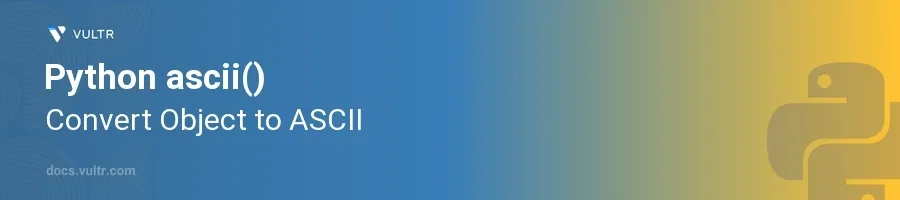
Introduction
The ascii()
function in Python is a built-in utility that converts any object into an ASCII string representation. This is particularly useful for rendering readable versions of objects that contain non-ASCII text, such as special characters from various languages, emoji, etc. The function ensures that these characters are represented in a way that can be printed and viewed in standard ASCII environments.
In this article, you will learn how to use the ascii()
function effectively across different data types. Explore practical examples to understand how ascii()
handles strings, lists, and custom objects, maintaining their readability across non-supporting ASCII environments.
Using ascii() with Strings
Handling Special Characters
Start with a string that includes non-ASCII characters.
Apply the
ascii()
function to this string.pythontext = "Pokémon Go" ascii_text = ascii(text) print(ascii_text)
This snippet converts the string
text
containing the non-ASCII character 'é' to its ASCII representation, displaying'Pok\\xe9mon Go'
.
Converting Emojis
Create a string containing emoji characters.
Use the
ascii()
function to convert these emojis to their ASCII representations.pythonemoji_text = "Hello, 😊!" ascii_emoji_text = ascii(emoji_text) print(ascii_emoji_text)
The code snippet converts the emoji '😊' to an escaped string format, resulting in
'Hello, \\U0001f60a!'
.
Applying ascii() to Collections
Using ascii() with Lists
Define a list containing a mix of ASCII and non-ASCII elements.
Convert the entire list to an ASCII string using
ascii()
.pythonmy_list = ['apple', 'café', 'schön'] ascii_list = ascii(my_list) print(ascii_list)
This approach ensures all characters, including 'é' and 'ö', are depicted in their ASCII-safe escaped form, such as
'['apple', 'caf\\xe9', 'sch\\xf6n']'
.
Handling Dictionaries
Construct a dictionary with non-ASCII keys and values.
Utilize the
ascii()
function to get an ASCII representation of the dictionary.pythonmy_dict = {'name': 'Björn', 'age': 28} ascii_dict = ascii(my_dict) print(ascii_dict)
This example displays keys and values in their ASCII-escaped format, ensuring compatibility across different environments.
Dealing with Custom Objects
Define a class with special characters in its representation.
Use the
ascii()
function on an instance of this class.pythonclass Product: def __init__(self, name, price): self.name = name self.price = price def __repr__(self): return f"Product(name='{self.name}', price={self.price})" p = Product('Café Noir', 5.99) print(ascii(p))
The
ascii()
conversion ensures that the product name 'Café Noir' is correctly escaped, resulting in a readable and ASCII-compatible representation of the object.
Conclusion
The ascii()
function is a versatile Python tool for ensuring that data, especially strings with non-ASCII characters, are represented in a way compatible with environments that only support ASCII characters. From handling special characters and emojis in strings to applying it across various data structures like lists and dictionaries, understanding ascii()
expands your ability to work with text data efficiently. This function is indispensable in data processing, logging, and situations where maintaining ASCII integrity is crucial. By following the examples provided, you equip yourself to handle diverse character sets gracefully in any Python project.
No comments yet.