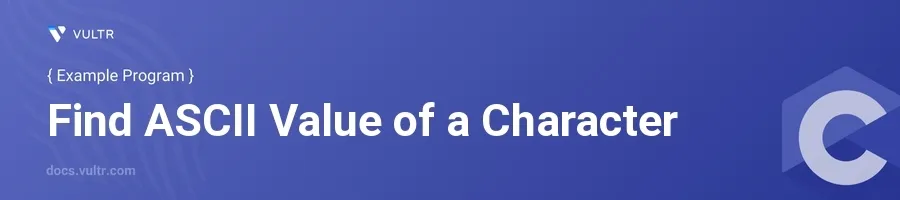
Introduction
When working with C programming, understanding ASCII values is fundamental, especially when dealing with character data types. ASCII, or American Standard Code for Information Interchange, is a character-encoding scheme used to represent text in computers. Every character on your keyboard is associated with a specific ASCII value, which is an integral number.
In this article, you will learn how to write a C program to find the ASCII value of a character. Explore multiple examples to solidify your understanding of how characters are translated into their corresponding ASCII values, improving your ability to manipulate and assess data effectively in your C programs.
Basic Program to Find ASCII Value
Output ASCII Value of a Single Character
Declare a character variable to store the input.
Use scanf to read a character from the user.
Print the ASCII value using printf.
c#include <stdio.h> int main() { char c; printf("Enter a character: "); scanf("%c", &c); printf("ASCII value of %c = %d", c, c); return 0; }
This code snippet creates a simple C program that reads a character from user input and prints its ASCII value. The
%c
format specifier inscanf
captures a character, and%d
inprintf
is used to print the integer value of the character, which corresponds to its ASCII value.
Advanced Usage: Loop Through Characters
Display ASCII Values for a Range of Characters
Initialize and control a loop to iterate over a defined range of characters.
Print the ASCII value for each character in the loop.
c#include <stdio.h> int main() { char c; for(c = 'a'; c <= 'z'; c++) { printf("ASCII value of %c = %d\n", c, c); } return 0; }
In this example, the program loops from
a
toz
and prints the ASCII value for each character. This is useful for understanding the sequence of ASCII values in a specific range, such as all lowercase alphabets.
Examine Non-Printable Characters
Find ASCII Values of Control Characters
Use a loop to iterate through ASCII values from 0 to 31, which are generally non-printable control characters.
Apply the
isprint()
function to filter non-printable characters and replace them with a placeholder for clear output.c#include <stdio.h> #include <ctype.h> int main() { int i; for (i = 0; i < 32; i++) { if(isprint(i)) { printf("ASCII value of %c = %d\n", i, i); } else { printf("ASCII value of non-printable character = %d\n", i); } } return 0; }
This code displays the ASCII values of control characters, which are generally not printable. The
isprint()
function fromctype.h
library is used to check if a character is printable. If not, it prints a generic message indicating the ASCII value belongs to a non-printable character.
Conclusion
Comprehending the ASCII value of characters in C enhances your ability to manipulate strings and characters effectively, providing a foundation for more complex text-processing tasks. Whether handling printable characters or diving deeper into non-printable control characters, your C programs can efficiently interact and process different types of character data. Use these examples as templates or starting points to expand your understanding and application of ASCII values in various programming scenarios.
No comments yet.