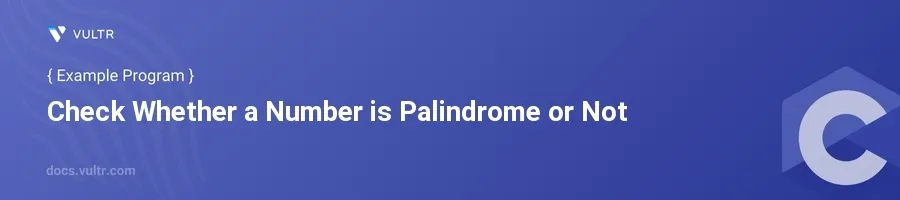
Introduction
A palindrome is a sequence that reads the same backward as forward. When dealing with numbers, this concept can be applied to identify whether a numerical value retains the same sequence of digits from either end. Detecting palindromes is a common task in programming challenges and can also be useful in certain math-based applications.
In this article, you will learn how to determine if a number is a palindrome in C programming language. Explore practical examples of how to implement this check efficiently, understand the logic behind the process, and discover best practices for structuring your code.
Determining Palindromes in C
Basic Approach: Reverse the Number
Read the original number.
Reverse the digits of the number.
Compare the reversed number with the original number.
c#include <stdio.h> int main() { int num, originalNum, reversedNum = 0, remainder; printf("Enter an integer: "); scanf("%d", &num); originalNum = num; // Reverse the number while (num != 0) { remainder = num % 10; reversedNum = reversedNum * 10 + remainder; num /= 10; } // Check if the original number is a palindrome if (originalNum == reversedNum) { printf("%d is a palindrome.\n", originalNum); } else { printf("%d is not a palindrome.\n", originalNum); } return 0; }
The given code prompts the user to enter an integer, reverses it, and compares the reversed number with the original. If they are the same, it concludes that the number is a palindrome.
Checking Halfway (Optimized Approach)
Extract half of the digits and reverse them.
Compare the reversed half with the other half (consider even and odd length numbers).
c#include <stdio.h> #include <stdbool.h> #include <stdlib.h> // For abs() function bool isPalindrome(int num) { int reversedHalf = 0; if (num < 0 || (num % 10 == 0 && num != 0)) { return false; } while (num > reversedHalf) { reversedHalf = reversedHalf * 10 + num % 10; num /= 10; } // Check for odd and even lengths return num == reversedHalf || num == reversedHalf / 10; } int main() { int num; printf("Enter an integer: "); scanf("%d", &num); if(isPalindrome(abs(num))) { printf("%d is a palindrome.\n", abs(num)); } else { printf("%d is not a palindrome.\n", abs(num)); } return 0; }
This code includes a function
isPalindrome
that determines if a number is a palindrome by comparing only half of it, improving efficiency particularly for very large numbers. It handles both even and odd-digit numbers and checks for negative numbers and edge cases like multiples of 10.
Conclusion
Checking if a number is a palindrome in C involves reversing the number and comparing it to the original or using optimized techniques for comparing only part of the number. The conventional method is straightforward but can be slow for large numbers, while the optimized approach improves performance by reducing the number of digits processed. Whichever method you choose, the fundamental steps involve modular arithmetic and integer manipulation, essential techniques in C programming. Begin implementing these methods for practical programming challenges and enhance your problem-solving skills in C.
No comments yet.