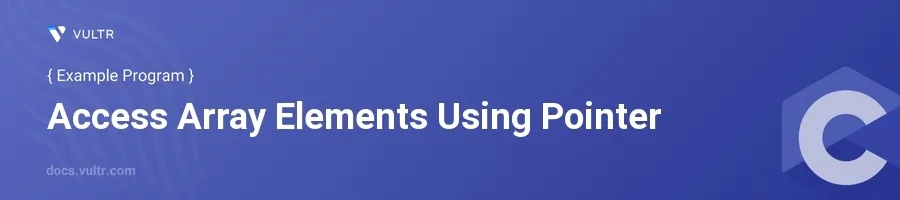
Introduction
Pointers in C programming language are a powerful feature, allowing direct memory access and manipulation. They are particularly useful when working with arrays, as they can be used to iterate over and manipulate array data efficiently. This capability proves essential in embedded systems, high-performance computing, and applications where direct control over memory allocation and processing is necessary.
In this article, you will learn how to access and manipulate array elements using pointers in C. Through detailed examples, discover how pointers operate with arrays, enabling both simple and complex data manipulations.
Accessing Array Elements with a Pointer
Basic Array Element Access
Define an array and a pointer.
Initialize the pointer to point to the array.
Access array elements via the pointer using array indexing.
c#include <stdio.h> int main() { int arr[5] = {10, 20, 30, 40, 50}; int *ptr = arr; for(int i = 0; i < 5; i++) { printf("Value at arr[%d] = %d\n", i, *(ptr + i)); } return 0; }
This code snippet defines an integer array
arr
and assigns its address to the pointerptr
. Inside the loop,*(ptr + i)
accesses thei
th element of the array. The pointer arithmetic moves the pointer from the start of the array to the required element.
Access Using Pointer Increment
Set a pointer to the start of the array.
Use a loop to increment the pointer itself to access each element.
c#include <stdio.h> int main() { int arr[] = {5, 15, 25, 35, 45}; int *ptr = arr; for(int i = 0; i < 5; i++) { printf("%d ", *ptr); ptr++; // Move pointer to next element } return 0; }
This method increments the pointer
ptr
directly, thereby accessing consecutive elements in the array. Each iteration prints the current value pointed to byptr
before moving the pointer to the next array element.
Modifying Array Elements Using Pointers
Change Values by Incrementing Pointers
Initialize the pointer to the start of the array.
Iterate over the array using the pointer and modify the array elements.
c#include <stdio.h> int main() { int nums[5] = {1, 2, 3, 4, 5}; int *ptr = nums; for(int i = 0; i < 5; i++) { *ptr *= 2; // Double each element printf("%d ", *ptr); ptr++; // Move to the next element } return 0; }
Each value in the array
nums
is doubled by dereferencing the pointer, modifying the element directly (*ptr *= 2
). As the pointer increments, it accesses and modifies each subsequent element of the array.
Conclusion
Utilizing pointers for array manipulation in C programming enhances both flexibility and efficiency. Whether reading or modifying array elements, pointers provide a mechanism for direct and effective memory operations. The examples discussed offer foundational techniques that can be built upon for more complex data handling tasks. Master these basic operations to harness the full power of pointers and arrays in your C programming projects.
No comments yet.