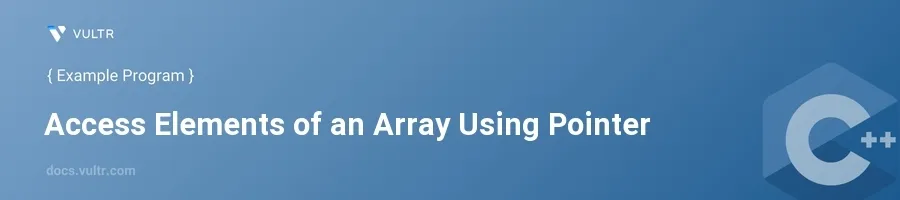
Introduction
Pointers in C++ are essential for efficient programming, especially when dealing with arrays. They allow direct memory access and manipulation, which can lead to more compact and faster code. This topic is particularly relevant in scenarios requiring low-level control of data storage, such as in systems programming or when optimizing performance-critical software.
In this article, you will learn how to access and manipulate elements of an array using pointers. You'll explore several examples that highlight different techniques and use cases, enhancing your understanding of pointers and arrays in C++.
Accessing Array Elements with Pointers
Basic Access and Navigation
Start by declaring an array and a pointer.
Point to the first element of the array using the pointer.
Access each element of the array through the pointer.
cpp#include <iostream> using namespace std; int main() { int numbers[] = {10, 20, 30, 40, 50}; int* ptr = numbers; // Pointer to the first element for(int i = 0; i < 5; ++i) { cout << *(ptr + i) << " "; } return 0; }
In this example,
ptr
points to the first element of the arraynumbers
. By incrementingptr
(i.e.,ptr + i
), you access subsequent elements of the array. The expression*(ptr + i)
dereferences the pointer to get the value at the corresponding array position.
Modifying Elements through Pointers
Initialize the pointer to point to the array as before.
Use the pointer to modify the elements of the array.
cpp#include <iostream> using namespace std; int main() { int numbers[] = {10, 20, 30, 40, 50}; int* ptr = numbers; for(int i = 0; i < 5; ++i) { *(ptr + i) = *(ptr + i) * 2; // Double each element } for(int i = 0; i < 5; ++i) { cout << numbers[i] << " "; } return 0; }
This code first doubles each element in the array
numbers
using the pointerptr
. Each element is accessed via*(ptr + i)
and modified directly. The second loop prints out the modified array to verify the changes.
Working with Multidimensional Arrays
Define a two-dimensional array and a pointer to its first element.
Use the pointer to traverse and manipulate the array.
cpp#include <iostream> using namespace std; int main() { int multi[2][3] = {{1, 2, 3}, {4, 5, 6}}; int* ptr = &multi[0][0]; // Pointer to the first element of multi for(int i = 0; i < 6; ++i) { cout << *(ptr + i) << " "; // Access every element linearly } return 0; }
This snippet assumes a linear traversal of a two-dimensional array using a single pointer. By calculating the index linearly,
ptr + i
moves through the entire 2D array as if it were a 1D array, proving the flexibility and power of pointers in array manipulations.
Conclusion
Mastering the use of pointers to access and manipulate array elements in C++ paves the way for writing more performant and lower-level code. From simple single-dimensional arrays to more complex multidimensional data structures, pointers provide a powerful toolset for direct memory manipulation. With the techniques discussed, you have the groundwork to apply these principles across a wide range of applications, enhancing both the efficiency and effectiveness of your C++ programs.
No comments yet.