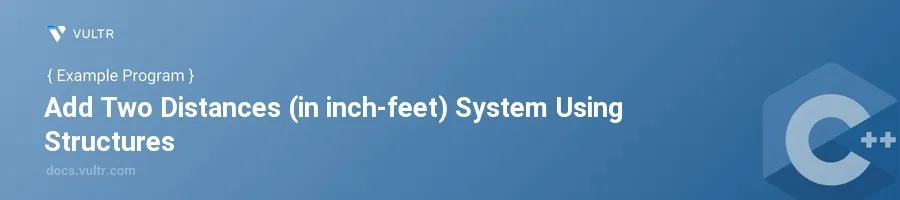
Introduction
Working with distances in C++ requires careful manipulation of units. When the distances are represented in feet and inches, maintaining accuracy and proper conversion between these units becomes crucial. Utilizing structures in C++ provides a systematic way to handle such data by grouping feet and inches into a single unit.
In this article, you will learn how to create a C++ program to add two distances, each expressed in inches and feet. Examine how structures facilitate the management of this data, ensuring that the program is both maintainable and extensible.
Defining a Structure for Distances
Create the Distance Structure
Define a structure named
Distance
that includes two members:feet
as an integer andinches
as a float.cppstruct Distance { int feet; float inches; };
Here,
Distance
acts as a custom data type to store distances with two separate components which arefeet
andinches
.
Initialize Distance Variables
Declare two variables of type
Distance
to store the distances you want to add.cppDistance dist1, dist2;
These variables,
dist1
anddist2
, will be used to input the two distances from the user and store them in terms of feet and inches.
Writing a Function to Add Distances
Implementing Add Distances Function
Create a function
addDistances
which takes two parameters of typeDistance
and returns aDistance
.cppDistance addDistances(Distance d1, Distance d2) { Distance sum; sum.inches = d1.inches + d2.inches; sum.feet = d1.feet + d2.feet + (int)(sum.inches / 12); sum.inches = fmod(sum.inches, 12); return sum; }
This function logically adds feet and inches from two
Distance
objects. It correctly handles the overflow of inches into feet using integral division and the modulo operation to separate inches.
Displaying the Result
Creating a function to display distance
Implement a function
printDistance
that takes aDistance
object and outputs the distance in feet and inches.cppvoid printDistance(Distance d) { cout << d.feet << " feet " << d.inches << " inches" << endl; }
printDistance
aids in the formal presentation of the distance in a clear and readable format.
Putting it All Together
Use both
addDistances
andprintDistance
functions within themain
function to display the result.cpp#include <iostream> #include <cmath> // For fmod() using namespace std; // Structure definition and function declarations... int main() { Distance dist1, dist2, result; // Assuming user inputs or predefined values dist1.feet = 5; dist1.inches = 8.5; dist2.feet = 3; dist2.inches = 11.2; result = addDistances(dist1, dist2); cout << "Total distance is: "; printDistance(result); return 0; }
Ensure that the structure definitions and functions are correctly included and verify that feet and inches are properly formatted and presented.
Conclusion
Learning to add distances using structures in C++ not only organizes the data effectively but also maintains clear separation of logic and presentation within the code. The presented techniques of managing feet and inch calculations can be adapted for other similar types of applications in C++. With structures providing clear data grouping, managing more complex data becomes streamlined, empowering you to maintain and extend your C++ applications efficiently.
No comments yet.