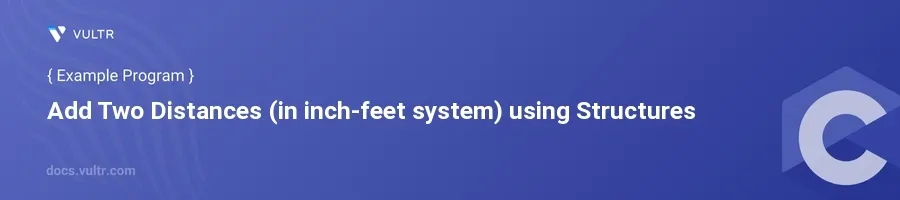
Introduction
A common application in programming is handling structured data to perform basic operations. In C, structures provide a way to group related variables of different types under a single name, making it easier to organize and manage data. When it comes to physical measurements like distances, structures can be particularly useful.
In this article, you will learn how to create and use structures in C to add two distances measured in inches and feet. This scenario underscores how structures can simplify operations on grouped data, such as arithmetic operations on distances.
Defining the Distance Structure
Create the Structure for the Distance
Begin by defining a structure called
Distance
that includes feet and inches as its members.c#include <stdio.h> typedef struct { int feet; float inches; } Distance;
This code block defines a new type
Distance
that has two members:feet
(an integer) andinches
(a float).
Adding Two Distance Values
Initialize and Input Distances
Declare two variables of type
Distance
and another one for storing the result.Ask the user to input the feet and inches for each distance.
cDistance dist1, dist2, result; printf("Enter information for 1st distance\n"); printf("Enter feet: "); scanf("%d", &dist1.feet); printf("Enter inches: "); scanf("%f", &dist1.inches); printf("Enter information for 2nd distance\n"); printf("Enter feet: "); scanf("%d", &dist2.feet); printf("Enter inches: "); scanf("%f", &dist2.inches);
Here,
scanf
is used to collect feet and inches for bothdist1
anddist2
from the user.
Perform the Addition of Two Distances
Write a function to add two
Distance
objects.Handle the conversion such that 12 inches make up one foot.
cDistance addDistances(Distance d1, Distance d2) { Distance result; result.inches = d1.inches + d2.inches; result.feet = d1.feet + d2.feet; if (result.inches >= 12.0) { result.inches -= 12.0; result.feet++; } return result; }
This function adds inches and feet separately and then checks if the total inches are equal to or greater than 12. If so, it converts 12 inches into 1 foot and adjusts the inches accordingly.
Output the Result
Call the addition function and display the result.
cresult = addDistances(dist1, dist2); printf("Sum of distances = %d\'-%.1f\"", result.feet, result.inches);
This completes the operation by displaying the sum of the two distances in feet and inches.
Conclusion
The use of structures in C to model real-world data such as distances is an effective way to maintain and manipulate related data. By creating a custom type, you simplify the processing of complex data and encapsulate related operations, such as the addition of distances in this example. By leveraging structures, your code becomes easier to manage and more modular, enhancing both readability and maintainability. Apply these techniques in your projects where grouping different but related data types is needed to enjoy organized and efficient code.
No comments yet.