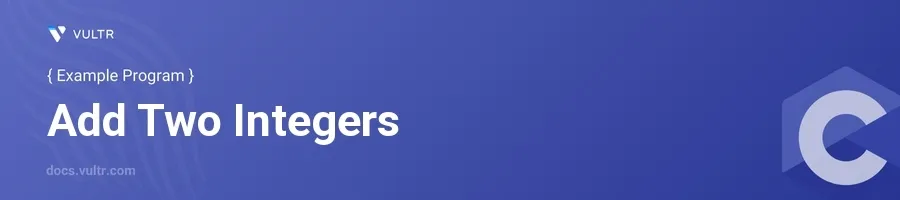
Introduction
Adding two integers is a fundamental operation in programming, often performed as one of the first tasks by beginners learning a new language. Demonstrating this task in C, a powerful and widely-used systems programming language, provides a clear insight into basic syntax and operations.
In this article, you will learn how to effectively add two integers using C programming. You will explore different examples that illustrate not only direct addition but also user interaction to receive input for the integers that need to be added.
Simple Addition of Two Integers
Example of Adding Two Known Integers
Declare two integer variables.
Assign values to these variables.
Add these integers and store the result.
Display the result.
c#include <stdio.h> int main() { int a = 10; int b = 20; int sum = a + b; printf("The sum of %d and %d is %d\n", a, b, sum); return 0; }
This snippet declares two integers
a
andb
, wherea
is assigned 10 andb
20. The addition of these numbers (a + b
) results insum
, which is then printed.
Asking Users for Input
Declare variables to store the integers and the sum.
Use
scanf
to allow user input for the integers.Add the integers.
Display the output.
c#include <stdio.h> int main() { int x, y, sum; printf("Enter two integers: "); scanf("%d %d", &x, &y); sum = x + y; printf("The sum of %d and %d is %d\n", x, y, sum); return 0; }
In this code,
x
andy
are input by the user viascanf
. The entered values are added and the sum is printed. The%d
format specifier is used for integer input and output.
Using Command Line Arguments for Input
Summation Using Arguments Passed to Main
Use the
argc
andargv
parameters of themain
function to handle command line arguments.Convert the string arguments to integers.
Perform the addition.
Print the result.
c#include <stdio.h> #include <stdlib.h> int main(int argc, char *argv[]) { if (argc != 3) { printf("Usage: %s num1 num2\n", argv[0]); return 1; } int num1 = atoi(argv[1]); int num2 = atoi(argv[2]); int sum = num1 + num2; printf("The sum of %d and %d is %d\n", num1, num2, sum); return 0; }
This program enables the addition of two numbers by accepting them as command line arguments. The
atoi
function converts the arguments from strings to integers. It checks if exactly two arguments are passed, otherwise, it provides usage information.
Conclusion
Adding two integers in C showcases the basics of variable declaration, user input, command line argument handling, and arithmetic operations. Each method described provides a different approach suited to specific scenarios, ranging from hardcoded values for simple demonstrations to dynamic input for interactive programs. Employing these techniques in various combinations lays a strong foundation for developing more complex C programs.
No comments yet.