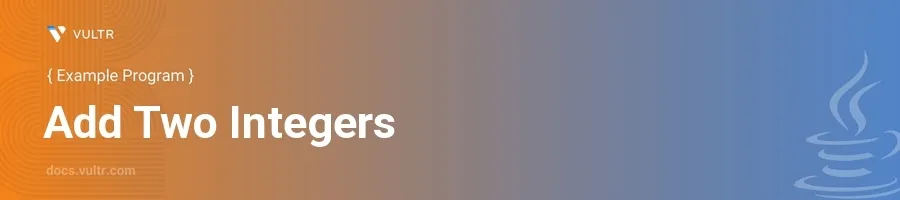
Introduction
In Java, one of the fundamental operations is adding two integers, which is an essential skill for any beginning programmer. This operation is often one of the first interactions new programmers have with arithmetic in coding, providing a clear example of how to manipulate data and utilize variables.
In this article, you will learn how to sum two integers in Java using different methods. The examples provided will not only show you the basic operation but also introduce variations for handling user input and using methods to perform the addition.
Addition Using Hard-Coded Values
Basic Example of Adding Two Integers
Define two integer variables with hard-coded values.
Sum the values and store the result in a third variable.
Print the result to the console.
javapublic class AddTwoNumbers { public static void main(String[] args) { int num1 = 5; int num2 = 10; int sum = num1 + num2; System.out.println("Sum of " + num1 + " and " + num2 + " is: " + sum); } }
This code snippet initializes two integers,
num1
andnum2
, with the values 5 and 10, respectively. It then calculates the sum of these two numbers and stores the result in the variablesum
. Finally, it prints out the result.
Addition Using User Input
Sum Two Integers Provided by the User
Import the Scanner class for taking input from the user.
Prompt the user to enter two numbers.
Read the entered values using the Scanner object.
Compute the sum and display it.
javaimport java.util.Scanner; public class AddIntegersUserInput { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter first integer:"); int firstInteger = scanner.nextInt(); System.out.println("Enter second integer:"); int secondInteger = scanner.nextInt(); int sum = firstInteger + secondInteger; System.out.println("The sum of " + firstInteger + " and " + secondInteger + " is " + sum); scanner.close(); } }
In this program,
Scanner
is used to collect two integers inputted by the user. It then calculates their sum and prints the result. Remember to close the Scanner object to prevent resource leaks.
Addition Using a Method
Create a Method to Add Two Numbers and Return the Result
Define a method named
addNumbers
that takes two integers as parameters and returns their sum.Call this method from the
main
method with user-defined or hard-coded values.javapublic class AddWithMethod { public static void main(String[] args) { int num1 = 3; int num2 = 7; int result = addNumbers(num1, num2); System.out.println("Sum is: " + result); } public static int addNumbers(int a, int b) { return a + b; } }
This example defines a static method
addNumbers
which performs the addition of two integers and returns the result.main
method calls this function with two integers, and the sum is then printed.
Conclusion
You can perform the addition of two integers in Java using several approaches, including hard-coding values, taking user input, or encapsulating the logic within a method. Each method helps in understanding different aspects of Java programming, from basic syntax and console IO to method creation and execution flow. Use these examples as a foundation to build more complex applications involving data manipulation and user interaction.
No comments yet.