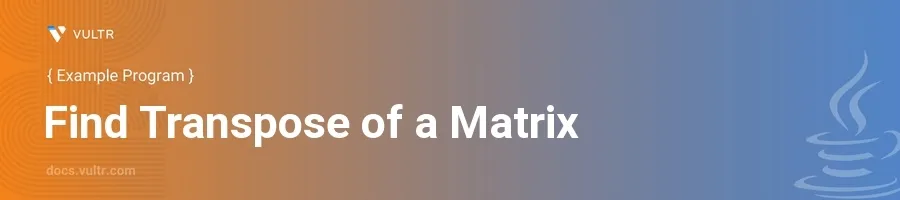
Introduction
In Java, the transpose of a matrix is obtained by swapping its rows with its columns. This process is commonly used in mathematical programming and data science for matrix manipulation. Understanding how to compute the transpose is essential for operations such as matrix multiplication, solving systems of linear equations, and many other applications in scientific computing.
In this article, you will learn how to write a Java program to find the transpose of a matrix. We will go through examples that demonstrate how to manipulate arrays to achieve this, adjusting rows and columns effectively.
Creating the Java Program
Define the Original Matrix
Begin by initializing and populating a 2D array which represents the matrix. Remember that in Java, a 2D array is essentially an array of arrays.
javaint original[][] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Here,
original
is a 3x3 matrix. Each inner array represents a row in the matrix.
Implement the Transpose Logic
To transpose the matrix, declare a new 2D array that will hold the transposed matrix.
Use nested loops to swap rows and columns.
javaint transpose[][] = new int[3][3]; for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { transpose[j][i] = original[i][j]; } }
This code iterates through each element of the
original
array, swapping the indices of the rows and columns to form thetranspose
array.
Display the Transposed Matrix
Print the transposed matrix to verify that the rows and columns have been swapped successfully.
javaSystem.out.println("Original Matrix:"); for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { System.out.print(original[i][j] + " "); } System.out.println(); } System.out.println("Transposed Matrix:"); for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { System.out.print(transpose[i][j] + " "); } System.out.println(); }
These loops go through each element of the
original
andtranspose
arrays, printing them in matrix format to the console. The output clearly illustrates the transformation from the original to the transposed matrix.
Handling Non-Square Matrices
Adapt the Program for Any Rectangular Matrix
Update the initialization of the original and transpose matrices to manage any size.
Adjust the loops to accommodate variable row and column lengths.
javaint original[][] = {{1, 2}, {3, 4}, {5, 6}}; int transpose[][] = new int[original[0].length][original.length]; // note the dimensions for (int i = 0; i < original.length; i++) { for (int j = 0; j < original[0].length; j++) { transpose[j][i] = original[i][j]; } } System.out.println("Non-Square Transposed Matrix:"); for (int i = 0; i < transpose.length; i++) { for (int j = 0; j < transpose[0].length; j++) { System.out.print(transpose[i][j] + " "); } System.out.println(); }
This example handles a non-square (rectangular) matrix, dynamically adjusting the transposition logic to fit matrices of varied dimensions.
Conclusion
Writing a Java program to compute the transpose of a matrix involves manipulating indices of a 2D array. By understanding how array indexing works in matrix operations, you can effectively transform, manipulate, and utilize matrices in your Java applications. From scientific computations to graphics transformations, these principles empower you to work more proficiently with multi-dimensional data structures. Ensure to adapt your transpose logic based on matrix dimensions, as seen in the examples, to accommodate both square and non-square matrices effectively.
No comments yet.