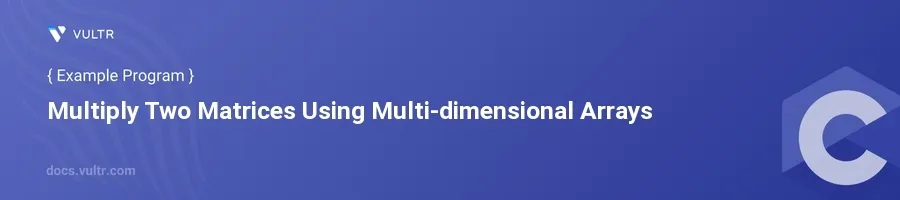
Introduction
Multiplying two matrices in programming commonly involves handling nested loops and manipulating array indices. In C, this specific task can leverage the structured syntax of the language—particularly its support for multi-dimensional arrays—to streamline operations on matrices.
In this article, you will learn how to develop a C program to multiply two matrices using multi-dimensional arrays. This guide will walk you through examples that demonstrate the step-by-step implementation of matrix multiplication, from declaring arrays to iterating over them to produce a new matrix.
Establishing Matrix Multiplication Logic
Define Matrices and Their Dimensions
Start by defining the dimensions of the matrices. Remember, for matrix multiplication to be possible, the number of columns in the first matrix must equal the number of rows in the second matrix.
Declare two 2D arrays for the matrices and another for the result.
c#include <stdio.h> int main() { int a[2][3] = {{1, 2, 3}, {4, 5, 6}}; int b[3][2] = {{7, 8}, {9, 10}, {11, 12}}; int result[2][2] = {0}; // Initialize all elements to 0 }
In this code, matrix
a
has dimensions 2x3, and matrixb
has dimensions 3x2, making the resulting matrix dimension 2x2.
Calculate the Product of Matrices
Use nested loops to calculate the product. The outer two loops iterate over each element of the resultant matrix, while the innermost loop performs the multiplication and sum required for each element in the resulting matrix.
Ensure the inner loop runs through the common dimension (columns of the first matrix or rows of the second matrix).
cint i, j, k; for (i = 0; i < 2; i++) { for (j = 0; j < 2; j++) { for (k = 0; k < 3; k++) { result[i][j] += a[i][k] * b[k][j]; } } }
This block of code iterates over each cell destined for the result matrix and calculates the sum of products for the respective position.
Output the Resulting Matrix
Print the resulting matrix to verify the correctness of the multiplication.
Loop through the result matrix and use
printf
to display the elements.cprintf("Resultant Matrix:\n"); for (i = 0; i < 2; i++) { for (j = 0; j < 2; j++) { printf("%d ", result[i][j]); } printf("\n"); } return 0;
The output loop goes through each element in the result matrix and prints it, formatting each row on a new line.
Conclusion
By following the steps and understanding the multidimensional array operations in C, you can effectively perform matrix multiplication. This tutorial provided fundamental knowledge and practical application to manipulate arrays and execute complex numerical operations. With these tools, expand and apply your skills to other matrix operations and scientific computing needs, enhancing your proficiency in managing data and calculations in C.
No comments yet.