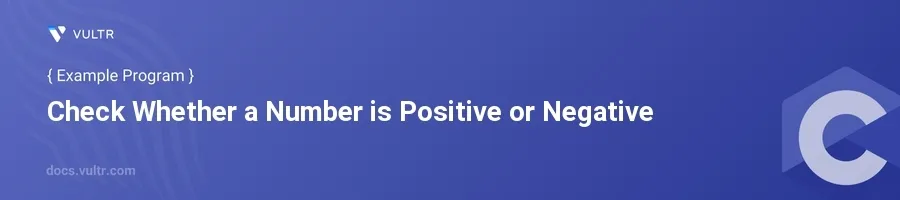
Introduction
Determining whether a number is positive or negative is a basic yet essential operation in programming. This functionality helps in decision-making processes such as conditional operations, loops, and more in various applications. It's fundamental in areas such as mathematics, physics simulations, and financial calculations, where the sign of a number affects computational results and logic flows.
In this article, you will learn how to implement a C program to check if a given number is positive or negative. You'll explore different examples that illustrate how to handle numerical input and make logical decisions based on its value.
Checking Number's Sign in C
Basic Positive or Negative Check
Prompt the user for input.
Use an
if-else
statement to determine if the number is positive or negative.Display the appropriate message based on the input.
c#include <stdio.h> int main() { int number; printf("Enter a number: "); scanf("%d", &number); if (number > 0) { printf("The number is positive.\n"); } else if (number < 0) { printf("The number is negative.\n"); } else { printf("The number is zero.\n"); } return 0; }
This program asks the user to input a number and checks if it is greater than, less than, or equal to zero. It then prints out whether the number is positive, negative, or zero.
Implementing with Logical Operators
Enhance understanding of conditional logic by introducing logical operators.
Use the combined conditions to refine the output messages.
c#include <stdio.h> int main() { int number; printf("Enter a number: "); scanf("%d", &number); if (number == 0) { printf("The number is zero.\n"); } else { printf("The number is %s.\n", (number > 0) ? "positive" : "negative"); } return 0; }
This version uses a ternary operator to simplify the decision-making process. The program checks whether the number is zero or not right away. If it's not zero, it further classifies the number as positive or negative using a ternary operation, which makes the code more concise.
Conclusion
Understanding how to check whether a number is positive or negative in C is a basic programming skill that has wide-ranging applications. The examples provided demonstrate straightforward methods using conditional statements and logical operators. Incorporate these techniques in your projects to efficiently handle numerical values and enhance your decision-making logic in your C programs. By mastering these approaches, ensure your code is not only functional but also clean and efficient.
No comments yet.