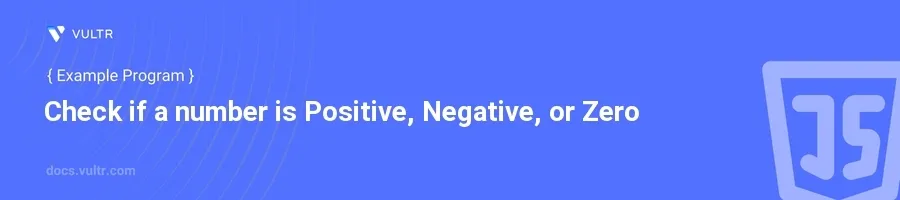
Introduction
Determining whether a number is positive, negative, or zero is a basic yet essential operation in many JavaScript programs. This task is usually one of the first encountered by beginning programmers and forms the foundation of more complex decision-making in code. Efficiently categorizing numbers can assist in directing the flow of operations based on numerical conditions.
In this article, you will learn how to implement a simple JavaScript function to check if a number is positive, negative, or zero. You'll explore several examples that demonstrate how to use conditional statements effectively in various scenarios involving numerical checks.
Implementing the Check
Basic Example with if-else
Statements
Define a function called
checkNumber
.Use
if-else
statements to determine the nature of the number.javascriptfunction checkNumber(num) { if (num > 0) { console.log(num + " is positive"); } else if (num < 0) { console.log(num + " is negative"); } else { console.log(num + " is zero"); } }
This code defines a function
checkNumber
that takes a single parameter,num
. It checks ifnum
is greater than, less than, or equal to zero, printing the appropriate message for each case.
Using Ternary Operator for Conciseness
Redefine the
checkNumber
function using the ternary operator for a more concise form.Employ nested ternary operators to handle all three conditions in one line.
javascriptfunction checkNumber(num) { let result = num > 0 ? num + " is positive" : num < 0 ? num + " is negative" : num + " is zero"; console.log(result); }
This variant uses nested ternary operators to compact the logic into a single line. It achieves the same result as the traditional
if-else
method but in a more concise manner.
Handling Non-numeric Inputs
Update the
checkNumber
function to first validate if the input is a number.Add an additional condition to handle non-numeric inputs gracefully.
javascriptfunction checkNumber(num) { if (typeof num !== 'number') { console.log("Please enter a valid number"); return; } if (num > 0) { console.log(num + " is positive"); } else if (num < 0) { console.log(num + " is negative"); } else { console.log(num + " is zero"); } }
In this updated function, there's a preliminary check to determine if the input
num
is actually a number. This is crucial for preventing runtime errors when the function is fed with inappropriate data types like strings orundefined
.
Conclusion
The ability to classify numbers as positive, negative, or zero in JavaScript is a fundamental skill that supports a range of more complex programming tasks. By developing this functionality through a simple JavaScript function, you enhance code modularity and reusability, while also ensuring robust data handling with type checking. Adapt these methods to fit into larger applications where such numerical evaluations are necessary, promoting cleaner and more efficient code across your projects.
No comments yet.