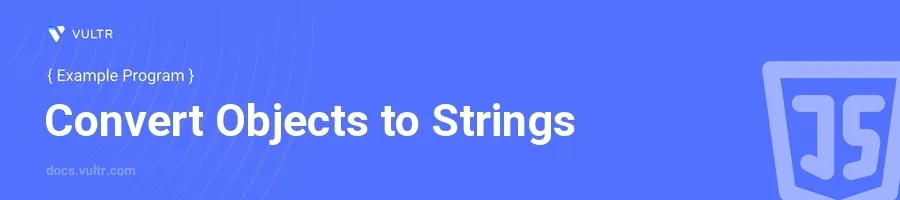
Introduction
In JavaScript, converting objects to strings is a common task, especially when logging, serializing, or combining information for display. Understanding various methods to perform this conversion allows for better data handling and debugging techniques in your applications.
In this article, you will learn how to convert JavaScript objects into strings using several different methods. Explore using JSON methods, custom functions, and JavaScript's built-in methods to achieve accurate and efficient conversions.
Using JSON.stringify
The JSON.stringify()
method is one of the most popular and straightforward ways to convert an object into a readable string format. It works not only with simple objects but also with arrays and nested objects.
Converting a Simple Object
Create an object with various key-value pairs.
Convert this object into a string using
JSON.stringify()
.javascriptlet person = { name: "Alice", age: 25, country: "USA" }; let stringifiedPerson = JSON.stringify(person); console.log(stringifiedPerson);
This code will output the string
{"name":"Alice","age":25,"country":"USA"}
. TheJSON.stringify()
function converts the JavaScript object into a JSON string.
Handling Nested Objects
Create a more complex object that includes nested objects.
Convert this nested object to a string.
javascriptlet personDetails = { name: "Bob", age: 30, address: { street: "123 Elm St", city: "Somewhere" } }; let stringifiedDetails = JSON.stringify(personDetails); console.log(stringifiedDetails);
This will convert the object, including the nested
address
object, into a string:{"name":"Bob","age":30,"address":{"street":"123 Elm St","city":"Somewhere"}
.
Using toString Method
Often, you might encounter objects where you want a custom string output rather than just JSON. JavaScript’s toString()
method can be customized in any object.
Customizing toString Method
Define an object with a custom
toString()
method.Use that method to convert the object to a string.
javascriptlet user = { name: "Charlie", age: 40, toString: function() { return `Name: ${this.name}, Age: ${this.age}`; } }; console.log(user.toString());
Executing this will display 'Name: Charlie, Age: 40'. The custom
toString()
method allows defining exactly how the object converts itself to a string.
Using Template Strings for Simple Conversions
For less complex scenarios where installing a full conversion isn't required, template strings (Template literals) can be adequate.
Using Template Strings
Produce an object with specifics.
Convert it to a string format manually using a template string.
javascriptlet product = { id: 101, name: "Coffee Maker" }; let productDescription = `Product [ID: ${product.id}, Name: ${product.name}]`; console.log(productDescription);
This produces:
Product [ID: 101, Name: Coffee Maker]
, a simple and readable string generated manually from the object properties.
Conclusion
Converting objects to strings in JavaScript is crucial for many reasons, ranging from debugging to data serialization. With methods like JSON.stringify()
, custom toString()
implementations, and even template literals, you have versatile tools at your disposal to handle various conversion needs efficiently. Utilize these methods depending on your specific requirements to effectively manage object-string conversion in your JavaScript projects.
No comments yet.