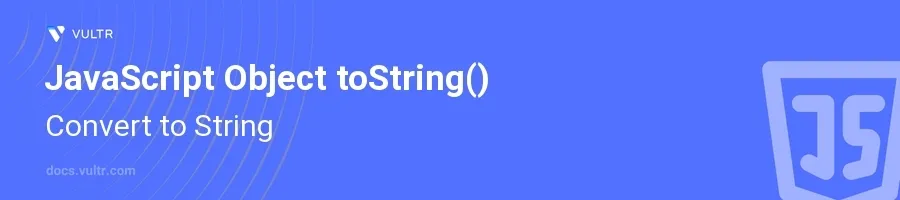
Introduction
The toString()
method in JavaScript is a powerful and versatile tool used to convert an object into a string representation. This method is built into the object prototype, meaning it's available for practically every object in JavaScript. It is particularly useful when debugging or when you need to display object properties in a readable format.
In this article, you will learn how to efficiently utilize the toString()
method across various JavaScript objects. Discover practical examples that demonstrate how to customize and handle string conversion, ensuring you can effectively integrate this functionality into your JavaScript projects.
Default Behavior of toString()
Understanding Basic Usage
Recognize that by default,
toString()
returns"[object Type]"
whereType
is the object type.Call the method on a built-in type.
javascriptconst obj = {}; console.log(obj.toString());
This outputs
"[object Object]"
, which is the default string representation for objects.
Apply toString() to Array Objects
Note that the default behavior for arrays is to concatenate all elements separated by commas.
Execute
toString()
on an array.javascriptconst arr = [1, 2, 3]; console.log(arr.toString());
In this case, it returns the string
"1,2,3"
.
Customizing toString()
Modifying toString() for Custom Objects
Understand that you can override the default
toString()
method.Define your own method for a custom object.
javascriptfunction Person(name, age) { this.name = name; this.age = age; } Person.prototype.toString = function() { return `Person(Name: ${this.name}, Age: ${this.age})`; }; const p = new Person("John", 30); console.log(p.toString());
This overrides the default behavior, returning a more descriptive string like
"Person(Name: John, Age: 30)"
.
Utilizing toString() with Inheritance
Be aware that if an object inherits from another, you can override
toString()
at different levels of the hierarchy.Create a subclass and override
toString()
.javascriptfunction Employee(name, age, jobTitle) { Person.call(this, name, age); this.jobTitle = jobTitle; } Employee.prototype = Object.create(Person.prototype); Employee.prototype.toString = function() { return `${Person.prototype.toString.call(this)} Job Title: ${this.jobTitle}`; }; const e = new Employee("Alice", 28, "Developer"); console.log(e.toString());
This method combines inherited properties with custom ones, resulting in a comprehensive string output.
Conclusion
The toString()
method in JavaScript offers extensive flexibility by allowing the conversion of objects to string representations either using the default or customized method. Employing this method enhances logging, debugging, and display of object properties. With the techniques discussed above, tailor the output of toString()
to provide detailed and relevant information, which can notably improve the user interface and development experience. Adapt your use of toString()
to fit your specific needs, ensuring your JavaScript code is both robust and user-friendly.
No comments yet.