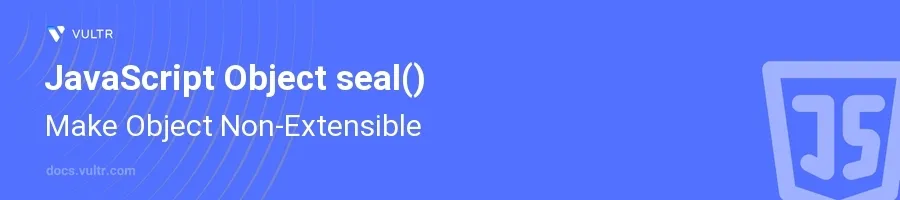
Introduction
The Object.seal()
method in JavaScript is a robust tool for controlling object modification. It allows you to seal an object, preventing new properties from being added to it while also marking all existing properties as non-configurable. Properties of a sealed object can still be changed as long as they are writable. This functionality is particularly useful in maintaining object integrity, avoiding accidental modifications or expansions after an object's initial creation and setup.
In this article, you will learn how to effectively use the Object.seal()
method to make objects non-extensible. Discover how to seal objects, check if an object is sealed, and understand the implications of sealing objects in your JavaScript projects.
Understanding Object.seal()
Sealing an Object
Start with a simple object.
Apply the
Object.seal()
function to restrict it.javascriptconst user = { name: "Alex", age: 30 }; Object.seal(user);
This code creaes an object
user
and seals it usingObject.seal()
. After sealing, you cannot add new properties to theuser
object, nor can you remove existing ones.
Modifying Properties After Sealing
Despite sealing, modify the values of existing writable properties.
javascriptuser.age = 31; console.log(user.age); // Outputs: 31
This example demonstrates modifying the age property of the sealed
user
object. The change is allowed because the existing properties remain writable.
Attempting to Extend the Sealed Object
Try to add a new property to the sealed object and observe the result.
javascriptuser.location = "New York"; console.log(user.location); // Outputs: undefined
Attempting to add a new property
location
to the sealeduser
object does not work, and accessinguser.location
returnsundefined
since the addition is ignored.
Checking if an Object is Sealed
Utilizing Object.isSealed()
Verify whether an object has been sealed.
javascriptconsole.log(Object.isSealed(user)); // Outputs: true
The
Object.isSealed()
method checks if the objectuser
is sealed, returningtrue
if it is sealed, andfalse
otherwise. This method is helpful for debugging and enforcing object integrity during runtime.
Implications of Sealing Objects
Understand the Key Effects of Sealing
- Prevents adding new properties.
- Sets all existing properties’ configurations to non-configurable.
Consider Use Cases
- Use
Object.seal()
when you want to lock an object's structure after its initial setup. - Use sealing to prevent future extensions or structural changes, especially when working with settings or configurations that must not be altered after loading.
Conclusion
The Object.seal()
method is a valuable tool for managing object mutability in JavaScript. By preventing extensions and making object properties non-configurable, you help ensure the stability and integrity of your objects throughout their lifecycle. Use Object.seal()
to maintain predictable object behavior, guard against inadvertent property additions or deletions, and uphold object structure as you develop and manage your JavaScript applications. Implementing these techniques secures your objects against modifications that could potentially disrupt your application’s state or behavior.
No comments yet.