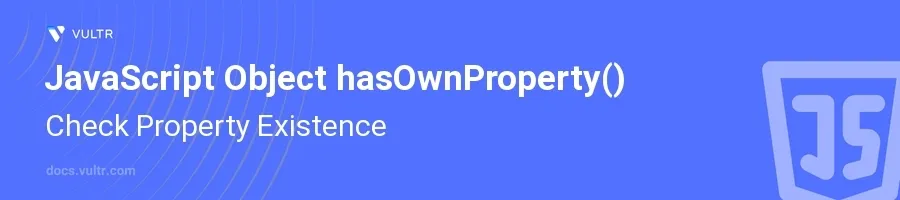
Introduction
The hasOwnProperty()
method in JavaScript is an essential function for checking whether an object contains a property as its own (not inherited from the prototype chain). This method is critical in ensuring that the properties you work with are indeed part of the object you're dealing with and not derived from its prototype. This provides a safer and more controlled handling of objects in your code.
In this article, you will learn how to use the hasOwnProperty()
method effectively across various scenarios. Explore how to verify the existence of properties directly within an object, thus differentiating them from those inherited through the prototype chain.
Usage of hasOwnProperty()
Check Direct Property of an Object
Define an object with properties.
Use
hasOwnProperty()
to determine if a specific property exists as a direct property of the object.javascriptvar car = {make: "Toyota", model: "Camry"}; var hasMake = car.hasOwnProperty('make'); var hasYear = car.hasOwnProperty('year'); console.log(hasMake); // Outputs: true console.log(hasYear); // Outputs: false
This example checks whether the
car
object has direct properties 'make' and 'year'. While 'make' is a property of the object (thus returningtrue
), 'year' is not (thus returningfalse
).
Use in a Function to Check Property
Craft a function to check if an object contains a given property as its own.
Pass the object and the property name as parameters.
Return the result of
hasOwnProperty()
.javascriptfunction checkProperty(obj, prop) { return obj.hasOwnProperty(prop); } var book = {title: "1984", author: "George Orwell"}; console.log(checkProperty(book, 'author')); // Outputs: true console.log(checkProperty(book, 'publisher')); // Outputs: false
Here, the function
checkProperty
verifies if 'author' and 'publisher' are direct properties of thebook
object. The function returnstrue
for 'author' andfalse
for 'publisher'.
Avoid Common Pitfalls with Prototype Inheritance
Understand that properties inherited from the prototype do not count as the object’s own properties.
Demonstrate the difference using an object created from a prototype.
javascriptfunction Vehicle(type) { this.type = type; } Vehicle.prototype.wheels = 4; var bike = new Vehicle("bike"); console.log(bike.hasOwnProperty('type')); // Outputs: true console.log(bike.hasOwnProperty('wheels')); // Outputs: false
Although 'wheels' is accessible on the
bike
object and returns the correct value, it is part of the prototype (Vehicle.prototype
) and not a direct property of thebike
object itself. Thus,hasOwnProperty('wheels')
returnsfalse
.
Conclusion
Utilizing the hasOwnProperty()
method in JavaScript ensures that you are working with properties that are an integral part of the object itself, not inherited from its prototype chain. This approach minimizes bugs and misunderstanding in your code especially when dealing with complex objects and inheritance. Implement the hasOwnProperty()
method to maintain clear and reliable object property checks in your applications, ensuring robust and predictable behavior in JavaScript applications.
No comments yet.