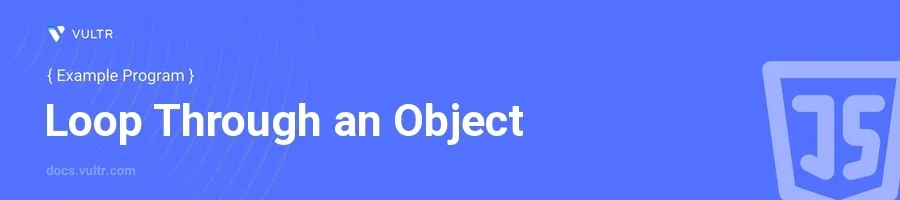
Introduction
Looping through an object in JavaScript is a fundamental skill that helps developers interact with and manipulate data stored in object structures. Since objects play a crucial role in representing real-world data within applications, understanding how to efficiently traverse them is essential for tasks like data processing, transformation, and display.
In this article, you will learn how to loop through properties of a JavaScript object using various methods, such as for...in
, Object.keys()
, and Object.entries()
. Each technique will be discussed with practical examples to illustrate how you can apply these strategies in your JavaScript projects.
Looping with the for...in Loop
The for...in
statement is one of the most straightforward methods for iterating over the properties of an object in JavaScript. It provides an easy way to access each key and therefore can be used to manipulate or review object data.
Retrieve and Display All Object Properties
Define an object with multiple properties.
Use a
for...in
loop to iterate over the object.javascriptconst person = { firstName: "John", lastName: "Doe", age: 30 }; for (let key in person) { console.log(key + ": " + person[key]); }
In this code, each property key in the
person
object is accessed through thefor...in
loop.console.log
is used to display each key-value pair.
Utilizing Object.keys()
Object.keys()
is a method that returns an array of a given object's own enumerable property names, iterated in the same order that a normal loop would. This can be paired with the forEach()
array method for effective results.
Print Each Property Value Using Object.keys()
Create an object with several properties.
Get all property keys using
Object.keys()
and useforEach()
to iterate over them.javascriptconst book = { title: "1984", author: "George Orwell", year: 1949 }; Object.keys(book).forEach(key => { console.log(book[key]); });
Here,
Object.keys(book)
generates an array of keys (['title', 'author', 'year']
), andforEach()
is used to loop through each key and print its corresponding value.
Employing Object.entries()
Object.entries()
method returns an array of a given object's own enumerable string-keyed property [key, value]
pairs. This is especially useful when accessing both the key and the value directly inside the loop.
Access Key-Value Pairs with Object.entries()
Define an object with properties.
Use
Object.entries()
andforEach()
for iterating.javascriptconst user = { username: "johndoe", email: "john.doe@example.com", verified: true }; Object.entries(user).forEach(([key, value]) => { console.log(`${key}: ${value}`); });
Object.entries(user)
gives an array of[key, value]
pairs, whichforEach()
iterates over, enabling direct access to both the key and value inside the loop.
Conclusion
Looping through an object in JavaScript can be accomplished using several effective methods, each suitable for different scenarios depending on the specific requirements of your project. Whether you need simple property access with for...in
, the array functionalities provided by Object.keys()
, or direct key-value pair handling via Object.entries()
, JavaScript offers the tools necessary to handle these tasks efficiently. Apply these looping techniques to enhance the way you work with objects in your JavaScript code, ensuring robust and flexible interaction with structured data.
No comments yet.