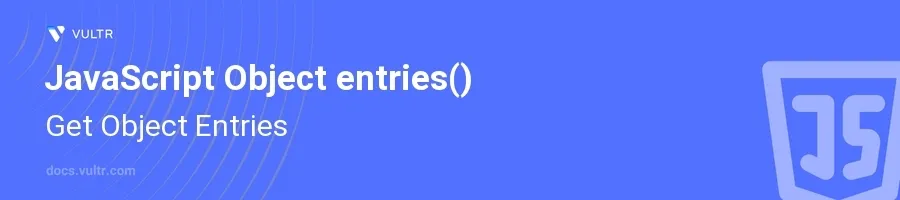
Introduction
The Object.entries()
method in JavaScript is a useful tool for accessing a list of a given object's own enumerable string-keyed property [key, value] pairs in the form of an array. This function simplifies the process of iterating over an object's properties, which is especially handy in situations that involve converting objects to arrays for easier manipulation or when pairing with other array methods.
In this article, you will learn how to effectively utilize the Object.entries()
method in different scenarios. Discover how this function can aid in data manipulation and transformation while exploring its integration with other JavaScript functionalities.
Basic Usage of Object.entries()
Retrieving Key-Value Pairs from an Object
Define an object with various properties.
Use
Object.entries()
to convert the object into an array of key-value pairs.javascriptconst object1 = { a: 'something', b: 42, c: false }; const entries = Object.entries(object1); console.log(entries);
This code snippet converts the object
object1
into an array of arrays, where each inner array is a [key, value] pair from the object.
Iterating Over Entries
Convert the object into an array of entries.
Use
forEach
to iterate over each entry.javascriptconst object1 = { a: 'something', b: 42, c: false }; Object.entries(object1).forEach(([key, value]) => { console.log(`${key}: ${value}`); });
In this example,
Object.entries()
is used to iterate over the object, and each key-value pair is logged to the console.
Integrating with Other Array Methods
Filter Object Entries
Use
Object.entries()
along withfilter()
to selectively remove entries based on a condition.javascriptconst object1 = { a: 'something', b: 42, c: false }; const filteredEntries = Object.entries(object1).filter(([key, value]) => typeof value === 'number'); console.log(filteredEntries);
Here,
filter()
is used to create a new array containing only the entries where the value is a number.
Transform Entries into a New Object
Map over entries and then reconstruct them into a new object.
Use
reduce()
to compile the mapped entries back into an object.javascriptconst object1 = { a: 'something', b: 42, c: false }; const transformedObject = Object.entries(object1) .map(([key, value]) => [key, value.toString()]) .reduce((acc, [key, value]) => ({ ...acc, [key]: value }), {}); console.log(transformedObject);
This snippet first converts each value to a string and then uses
reduce()
to build a new object with these transformed entries.
Conclusion
The Object.entries()
method in JavaScript is a versatile function that allows for easy transformation and iteration over object properties. By converting objects into arrays of entries, you can leverage array methods like filter()
, map()
, and reduce()
to manipulate and process data effectively. Implement these techniques in your JavaScript projects to handle objects dynamically and efficiently, enhancing both the readability and functionality of your code.
No comments yet.