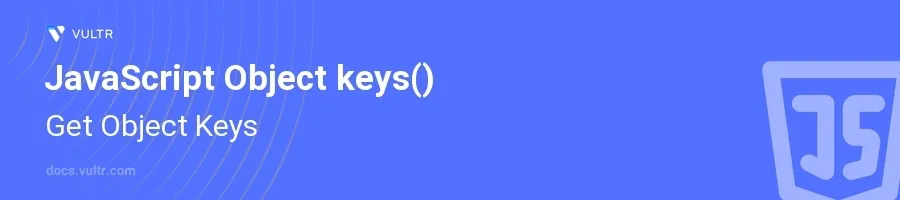
Introduction
The Object.keys()
method in JavaScript is a handy tool for extracting the keys from an object. This method is pivotal when you need to manipulate or iterate over an object's properties, enabling efficient data manipulation and retrieval within your applications.
In this article, you will learn how to effectively utilize the JavaScript Object.keys()
method in various programming scenarios. Explore how this method facilitates operations on objects, especially when dealing with dynamic property names and values.
Understanding Object.keys() in JavaScript
Retrieve Keys from a Simple Object
Define a basic object with various properties.
Use
Object.keys()
to extract the properties into an array.javascriptconst person = { name: "Alice", age: 25, occupation: "Developer" }; const keys = Object.keys(person); console.log(keys);
This code snippet extracts the keys
name
,age
, andoccupation
from theperson
object and stores them in thekeys
array, outputting['name', 'age', 'occupation']
.
Using Object.keys() with Complex Objects
Consider an object with nested properties.
Apply
Object.keys()
to access the top-level keys.javascriptconst user = { id: 1, profile: { username: "tech_guru", email: "example@mail.com" }, isActive: true }; const topLevelKeys = Object.keys(user); console.log(topLevelKeys);
In this example,
Object.keys()
retrieves the keysid
,profile
, andisActive
from theuser
object. If you're looking for a way to efficiently extract property names, usingjs get key
of object withObject.keys()
is a straightforward approach.
Iteration over Object Keys
Use
Object.keys()
to iteratively process all keys in an object.Combine it with methods like
Array.forEach()
for operations on each key.javascriptconst settings = { volume: 10, brightness: 70, mode: "night" }; Object.keys(settings).forEach(key => { console.log(`Key: ${key}, Value: ${settings[key]}`); });
Here, the keys from the
settings
object are iterated over, and for each key, both the key and its corresponding value are printed.
Using Object.keys() in Practical Scenarios
Filter Properties by Key Names
Utilize
Object.keys()
to filter object properties based on a condition.Implement conditional filtering within the iteration.
javascriptconst data = { a1: "hello", a2: "world", b1: "welcome", b2: "Earth" }; const filteredKeys = Object.keys(data).filter(key => key.startsWith('a')); console.log(filteredKeys);
The
filter()
function, combined withstartsWith()
, helps in selecting keys that begin with the letter 'a'.
Combine with Object Destructuring for Selective Retrieval
Work with
Object.keys()
alongside destructuring to selectively extract multiple properties.Integrate these techniques to enhance readability and efficiency.
javascriptconst personDetails = { firstName: "John", lastName: "Doe", age: 30, occupation: "Engineer" }; const selectedKeys = ['firstName', 'lastName']; const selectedData = Object.fromEntries( Object.entries(personDetails).filter(([key]) => selectedKeys.includes(key)) ); console.log(selectedData);
This approach utilizes destructuring and
Object.entries()
to filter and recreate an object that only contains thefirstName
andlastName
properties.
Conclusion
The Object.keys()
function in JavaScript offers a streamlined way to access the keys of an object, enriching the ability to handle and manipulate data dynamically. By exploring the various methods of leveraging this function, you can enhance data management tasks in your applications. With the techniques discussed, your JavaScript code becomes more flexible and powerful in dealing with objects, from simple key retrieval to more complex data structuring and filtering.
No comments yet.