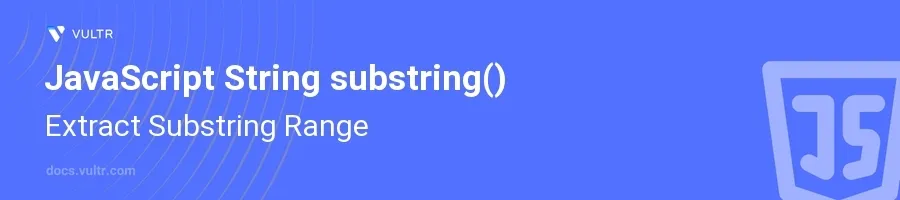
Introduction
The substring()
method in JavaScript is essential for extracting parts of a string based on specified index positions. It provides flexibility in string manipulation, particularly useful in text processing, data formatting, and programming tasks requiring substring extraction according to dynamic conditions.
In this article, you will learn how to efficiently use the substring()
method to extract string ranges. Understand how to specify start and end index parameters and explore common use cases, such as extracting a date from a timestamp or characters from a user-generated input.
Using substring() to Extract a Range
Basic Syntax of substring()
Grasp the primary syntax of
substring()
and how indices work.Use
substring()
by providing the start and end indices for the range you want to extract.javascriptvar fullString = "Hello, world!"; var partString = fullString.substring(7, 13); console.log(partString);
This script outputs
world
, extracted from thefullString
using thesubstring()
method. The extraction starts at index 7 and ends just before index 13.
Understanding Index Parameters
Recognize that the first parameter is the start index and the second is the end index, which is not inclusive.
Note that if the end index is omitted,
substring()
extracts till the end of the string.javascriptvar text = "JavaScript Guide"; var segment = text.substring(4); console.log(segment);
Here, the output is
Script Guide
as the extraction starts from index 4 and goes to the end of the string.
Handling Out-of-Range Indexes
Understand that JavaScript handles out-of-bounds indices by treating too small values as 0 and ignoring too large values.
javascriptvar message = "Hello, world!"; var extracted = message.substring(-1, 15); console.log(extracted);
The output is
Hello, world!
despite the intentional use of an out-of-range negative index.
Swapping Indices Automatically
Learn that if the start index is greater than the end index,
substring()
will swap them automatically.javascriptvar phrase = "Good Day"; var subphrase = phrase.substring(5, 2); console.log(subphrase);
The result is
od D
, demonstrating howsubstring()
adjusts the indices to readsubstring(2, 5)
internally.
Practical Examples of Using substring()
Extracting a Date from a Timestamp
Use
substring()
to extract date from a standard timestamp format.javascriptvar timestamp = "2023-09-15T12:05:30Z"; var date = timestamp.substring(0, 10); console.log(date);
This code captures just the date portion
2023-09-15
from a full timestamp.
Retrieving Usernames from Email Addresses
Extract the part of an email address before the "@" symbol.
javascriptvar email = "example@domain.com"; var username = email.substring(0, email.indexOf("@")); console.log(username);
The script fetches the user's name
example
by cutting off at the "@" character's index.
Conclusion
The substring()
method in JavaScript serves as a powerful tool for extracting specified ranges from strings. Its intuitive handling of indices, including adjustments for out-of-bounds values, makes string manipulation tasks straightforward and error-resistant. Apply this method in diverse scenarios, from formatting strings to complex data parsing, to achieve clean and efficient code outcomes. By mastering substring()
, you enhance the text processing capabilities of your JavaScript projects.
No comments yet.