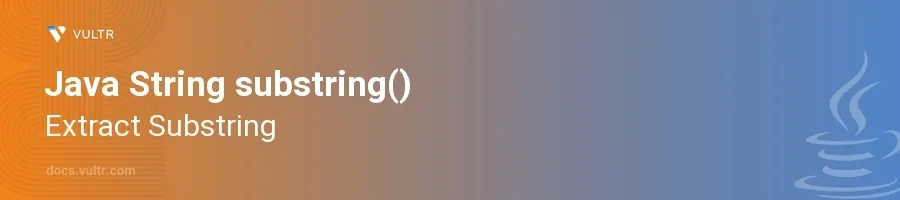
Introduction
The substring()
method in Java is a fundamental tool for string manipulation, allowing developers to extract specific parts of a string based on given index positions. This method is incredibly useful for tasks such as parsing data, cleaning inputs, and preparing text for further processing or display.
In this article, you will learn how to efficiently use the substring()
method in Java. This guide covers different ways to apply this method, ensuring you can adapt its use to various scenarios and understand the handling of edge cases.
Understanding the substring() Method
The substring()
method in Java is available in two variations, each serving to extract parts of a string based on different sets of parameters:
substring(int beginIndex)
: This version takes a single argument, the starting index, and returns a substring from this index to the end of the string.substring(int beginIndex, int endIndex)
: This version takes two arguments, the starting index and the ending index, and returns a substring starting at thebeginIndex
and extending up to, but not including, theendIndex
.
Basic Usage of substring()
To extract a substring from a given string using only the starting index:
javaString str = "Hello World"; String result = str.substring(6); System.out.println(result);
This snippet extracts the substring starting from index '6' to the end of the string
str
, which results in "World".To extract a substring using both the starting and ending indices:
javaString str = "Hello World"; String result = str.substring(0, 5); System.out.println(result);
Here, the substring starting at index '0' and ending before index '5' is extracted, resulting in "Hello".
Handling Exceptions with substring()
It is essential to handle potential exceptions that might arise from inappropriate index values:
IndexOutOfBoundsException
can occur if eitherbeginIndex
is negative,endIndex
is larger than the string's length, orbeginIndex
is greater thanendIndex
.javatry { String str = "Hello World"; String result = str.substring(12); // This line will throw an exception System.out.println(result); } catch (IndexOutOfBoundsException e) { System.out.println("Index is out of bounds: " + e.getMessage()); }
This code block illustrates handling an exception when the provided index is beyond the string's length.
Advanced Scenarios Using substring()
Extracting Substrings in Loops
Looping over a string to extract multiple substrings is a common use case, for example, parsing a formatted data string:
Consider a scenario where you want to extract words separately from a string containing space-separated words:
javaString sentence = "Java substring method"; String[] words = sentence.split(" "); for(String word : words) { System.out.println(word); }
Here, you first split the string into words and then could use
substring()
if more specific text manipulation was needed inside the loop.
Using substring() with Other String Methods
Combining substring()
with other string methods can powerfully refine text processing capabilities:
Suppose you need to remove white space and extract a specific part of a string:
javaString str = " Hello Java "; String trimmedAndSub = str.trim().substring(6); System.out.println(trimmedAndSub);
This code first removes leading and trailing spaces with
trim()
and then extracts the substring beginning at index '6' of the trimmed string, outputting "Java".
Conclusion
The substring()
method in Java is a versatile tool that enhances text manipulation capabilities. Master the use of this method to handle strings in your Java applications more effectively. Through the guided examples and scenarios provided, harness the power of string manipulation to develop cleaner, more efficient Java code. Manage strings with confidence, extract necessary parts, and combine substring()
with other methods to address complex string handling tasks.
No comments yet.