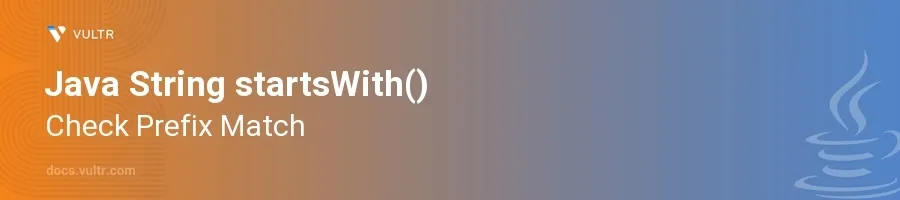
Introduction
The startsWith()
method in Java's String
class is essential for checking whether a particular string begins with a specified prefix. This method is widely used in parsing and validating data in applications where prefix accuracy determines the flow of execution or data classification.
In this article, you will learn how to utilize the startsWith()
method effectively across different use cases. Explore how to implement this function in your Java applications to check prefixes in strings, enhance the capabilities of data filtering, and make your code more organized and efficient.
Understanding the startsWith() Method
Basic Usage of startsWith()
Begin by creating a basic string that you want to check.
Apply the
startsWith()
method to determine if the string begins with the specified prefix.javaString filename = "example.txt"; boolean isTextFile = filename.startsWith("example"); System.out.println("Is Text File: " + isTextFile);
This example checks if the filename "example.txt" starts with the prefix "example". The output will be
true
because "example.txt" does indeed start with "example".
Case Sensitivity in Checking
Understand that
startsWith()
is case-sensitive.Create a scenario where case sensitivity is crucial for accurate data handling.
Execute the
startsWith()
method to observe the case-sensitive behavior.javaString greeting = "Hello World"; boolean correctGreeting = greeting.startsWith("Hello"); boolean incorrectGreeting = greeting.startsWith("hello"); System.out.println("Correct greeting: " + correctGreeting); System.out.println("Incorrect greeting: " + incorrectGreeting);
In this code snippet,
correctGreeting
will betrue
, andincorrectGreeting
will befalse
since "Hello" and "hello" are perceived differently due to case sensitivity.
Advanced Uses of startsWith()
Checking with Offset
Sometimes, you need to verify a prefix in a string from a particular offset.
Use the overloaded version of
startsWith()
that accepts an offset.javaString storagePath = "images/example.png"; boolean isImage = storagePath.startsWith("example", 7); System.out.println("Is Image File: " + isImage);
Here, the method checks if "storagePath" starts with "example" at index 7. The method returns
true
because starting from the 7th position in "images/example.png", the substring "example" matches.
Use in Conditional Structures
Integrate
startsWith()
in control structures likeif-else
to direct program flow based on string prefix.javaString url = "https://example.com"; if (url.startsWith("https")) { System.out.println("Secure connection"); } else { System.out.println("Insecure connection"); }
This example uses
startsWith()
to check the protocol of a URL. It prints "Secure connection" because the URL starts with "https", indicating an HTTPS connection.
Real-world Application
Apply
startsWith()
in real-world scenarios, such as file extension checking or protocol validation.Implement a simple method to classify files based on their extensions.
javaString document = "report.docx"; if (document.startsWith("report")) { System.out.println("This is a report file"); } else { System.out.println("Unknown file type"); }
This function verifies if the file name "document" starts with "report", efficiently classifying the file if it matches the prefix.
Conclusion
The startsWith()
method in Java is a powerful tool for prefix evaluation in strings. It allows for straightforward checks and logical decisions based on the beginning substrings. By mastering its use, especially with the capability to specify an offset, you enhance the flexibility and robustness of your string handling within Java applications. Apply these techniques to improve data validation, file handling, and URL processing in your projects, ensuring your code is both efficient and easily maintainable.
No comments yet.