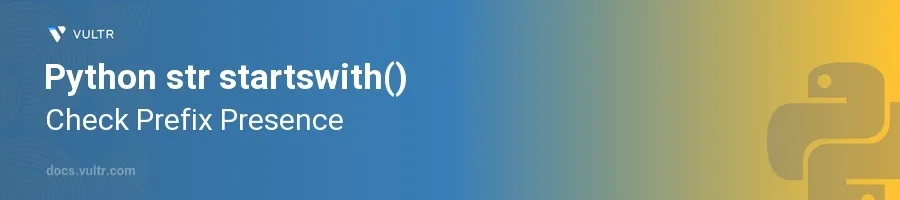
Introduction
The startswith()
method in Python is a string method that checks whether a given string starts with a specified prefix. This check is case-sensitive and plays a critical role in data validation, parsing, and filtering tasks when dealing with textual data. Understanding how to effectively use this method can greatly simplify code when conditions based on string prefixes are required.
In this article, you will learn how to leverage the startswith()
method in several practical scenarios. Explore methods to verify prefixes in strings, enhance data filtering, and apply conditional logic based on textual patterns. Harness the capabilities of startswith()
to improve string manipulation and data processing tasks.
Starting with Basic Prefix Checks
Checking a Simple Prefix
Create a baseline string that you will check against.
Define a prefix you expect the string to start with.
Use the
startswith()
method to verify the presence of this prefix.pythonbasic_string = "Hello, World!" prefix = "Hello" result = basic_string.startswith(prefix) print(result)
This code checks if
basic_string
starts with the prefix "Hello", and since it does,startswith()
returnsTrue
.
Using Prefix Checking for Data Validation
Prepare a list of user inputs that need verification.
Specify acceptable prefixes for these inputs.
Apply
startswith()
to ensure each input begins appropriately before further processing.pythonuser_inputs = ["username: John", "email: john@example.com", "password: 123456"] valid_prefix = "username" filtered_inputs = [user for user in user_inputs if user.startswith(valid_prefix)] print(filtered_inputs)
Here, only entries starting with the "username" prefix are selected. This helps in filtering out relevant data from a list of mixed types of inputs.
Handling Multiple Prefix Options
Checking Multiple Prefixes
Define a string to perform checks on.
Create a tuple containing multiple allowed prefixes.
Use
startswith()
with the tuple to check for any of the prefixes.pythonquery = "SELECT * FROM users" prefixes = ("SELECT", "INSERT", "UPDATE") check = query.startswith(prefixes) print(check)
This snippet checks if
query
starts with any SQL command types specified inprefixes
. If it matches one, it returnsTrue
.
Using the Start Position Parameter
Understand that
startswith()
can also check for prefixes starting from a specific index of the string.Define your string and the prefix to check.
Set the position from which the prefix checking should commence.
pythonmessage = "Error: Invalid user input" error_prefix = "Error" result = message.startswith(error_prefix, 0, 5) # Check between index 0 and 5 print(result)
In this case, the method checks if
message
starts with "Error" within the first five characters. Given the position and range, it returnsTrue
.
Practical Applications in Data Processing
Filtering Log Files for Specific Entries
Simulate opening a log file and reading lines that represent log entries.
Declare significant event types to search with prefixes.
Extract lines that begin with those event types using
startswith()
.pythonlog_entries = ["Error: Connection failed", "Warning: Low disk space", "Info: Update completed"] important_types = ("Error", "Warning") important_logs = [entry for entry in log_entries if entry.startswith(important_types)] print(important_logs)
Each log entry is evaluated, and entries starting with "Error" or "Warning" are selected. This method helps filter critical log information effectively.
Conclusion
The startswith()
method in Python is a robust tool for evaluating if strings meet specific prefix criteria. Employ this method to simplify initial checks on data, ensure robust data validation, and filter contents based on textual beginnings. Through various examples, understand how to enhance your Python projects by integrating conditional logic that relies on prefix validation efficiently. By mastering startswith()
, you elevate the reliability and readability of your Python code, particularly in scenarios involving extensive string manipulation.
No comments yet.