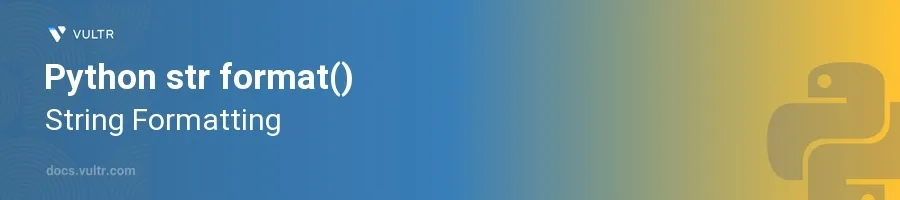
Introduction
The format()
method in Python is a powerful tool for string formatting, allowing developers to create formatted strings dynamically. It makes code cleaner and more readable by providing a way to interpolate data into strings. The format()
method can handle complex formatting operations with ease, including padding, alignment, and numerical formatting, which are typical in data reporting and user output generation.
In this article, you will learn how to apply the format()
method across various string formatting scenarios. Dive into simple text scenarios, numerical data formatting, and alignment techniques to enhance the way your program handles and presents information.
Basic Usage of format()
Simple Text Formatting
Define a base string with placeholders using curly braces
{}
.Pass values through the
format()
method to replace placeholders.pythonbase_string = "Hello, {}!" formatted_string = base_string.format("Alice") print(formatted_string)
This example injects the name "Alice" into the placeholder in
base_string
. The result is "Hello, Alice!".
Formatting Multiple Values
Create a string with multiple placeholders.
Supply multiple arguments to
format()
corresponding to the placeholders.pythontemplate = "{}, you have {} unread messages." result = template.format("Alice", 5) print(result)
Here, "Alice" replaces the first placeholder, and
5
replaces the second, resulting in "Alice, you have 5 unread messages."
Formatting Numerical Data
Formatting Integers
Insert placeholders for integers in your string.
Use colon
:
inside the curly braces to specify format codes liked
for integers.pythontext = "The number is: {:d}" formatted = text.format(123) print(formatted)
The
{:d}
format specifies an integer, so123
is formatted as "The number is: 123".
Decimal Precision for Floats
Use format specifiers to control the precision of decimal numbers.
Specify the desired precision with
.2f
for two decimal places.pythonstatement = "The value of pi is approximately {:.2f}" formatted_statement = statement.format(3.14159) print(formatted_statement)
This formats the floating-point number
3.14159
to two decimal places, resulting in "The value of pi is approximately 3.14".
Advanced Formatting with Alignment
Left Alignment
Apply left alignment to text using
<
within the format specifier.Specify the width to determine the field size in which text aligns.
pythonleft_aligned = "{:<10} is a scientist." formatted_text = left_aligned.format("Alice") print(formatted_text)
The output "Alice is a scientist." shows
Alice
aligned to the left within a 10-character field.
Center and Right Alignment
Use
>
for right alignment and^
for center alignment.Mention the width to align the text within a fixed space.
pythonright_aligned = "{:>10} arrived." centered_text = "{:^10} was here." print(right_aligned.format("Bob")) print(centered_text.format("Alice"))
Bob
gets right-aligned andAlice
gets centered in their respective 10-character fields.
Conclusion
The format()
function in Python unlocks significant potential in string manipulation, providing robust formatting options that are essential for producing clear and professional output. Whether handling simple text or complex numerical data, you achieve a high level of control over how information is presented. Utilize the formatting capabilities discussed to improve legibility and design of data outputs in your Python projects, ensuring your applications communicate effectively with users.
No comments yet.