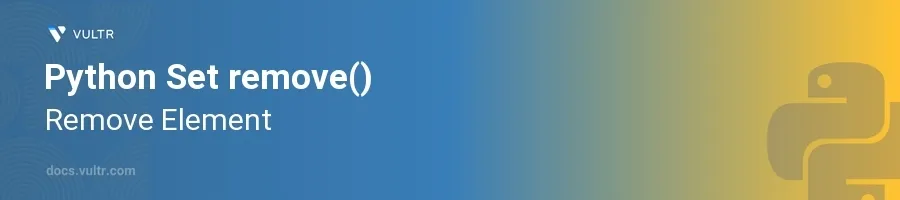
Introduction
The remove()
method in Python's set data structure allows for the removal of a specific element from a set. This function is significant because sets are commonly used for storing unique items, and managing the contents dynamically can be crucial for maintaining data integrity and efficiency in your code.
In this article, you will learn how to utilize the remove()
method in various applications involving sets. Discover how to effectively manipulate set contents, handle exceptions gracefully, and apply this method in practical scenarios such as data filtering and cleanup operations.
Utilizing the remove() Method
Basic Removal Operation
Initialize a set with multiple elements.
Apply the
remove()
method to take out a specific element.pythonmy_set = {1, 2, 3, 4, 5} my_set.remove(3) print(my_set)
This code removes the element
3
frommy_set
. The updated set no longer contains the number 3. It's a direct way to modify the set without leaving any trace of the removed element.
Handling Non-existent Elements
Understand that trying to remove an element that doesn't exist in the set will raise a
KeyError
.Introduce error handling using
try-except
to manage attempts to remove non-existent elements gracefully.pythonmy_set = {1, 2, 4, 5} try: my_set.remove(3) except KeyError: print("Element not found in the set.")
In this case, because the number
3
is not a member ofmy_set
, theremove()
method triggers aKeyError
. The except block catches this exception and prints a message, thus avoiding a program crash and handling the error effectively.
Using remove() in Loop Operations
Utilize looping constructs to conditionally remove items from a set.
Iterate through a copy of the set while attempting to remove items to prevent modifying the set during iteration.
pythonmy_set = {1, 2, 3, 4, 5, 6} for item in list(my_set): if item % 2 == 0: my_set.remove(item) print(my_set)
Here, the loop iterates over a list copy of
my_set
and removes each even number. The resulting set contains only odd numbers. Looping over a copy is crucial as it prevents runtime errors associated with changing the set size during iteration.
Practical Applications of remove()
Data Cleanup
Removing unwanted or duplicate data from a dataset often involves set operations. Using remove()
can help in filtering out unnecessary elements.
Create a set with duplicate and unnecessary entries.
Use the
remove()
method selectively to clean up the set.pythondata_set = {"data", "info", "data", "value", "sample"} unwanted = {"data", "sample"} for item in unwanted: data_set.remove(item) print(data_set)
After executing this snippet,
data_set
is left with elements that are deemed necessary. This approach is straightforward for maintaining high-quality data pools.
Synchronizing Data
In scenarios where two sets are being used to maintain synchronized data, elements may need to be removed from one set based on the contents of another.
Manage two sets representing different data stores.
Remove elements from one set based on the intersection with another set.
pythonprimary_set = {1, 2, 3, 4, 5} reference_set = {2, 3} for item in reference_set: primary_set.remove(item) print(primary_set)
This process ensures that
primary_set
only contains items that are not present inreference_set
, useful for maintaining exclusive data sets without overlap.
Conclusion
The remove()
method in Python sets is a fundamental tool for dynamically managing the elements within a set. It allows for direct, precise modifications to the set, and with proper handling of exceptions, it ensures that your code remains robust and error-free. Use the remove()
method to maintain clean data sets, synchronize contents between data structures, and perform many other data manipulation tasks efficiently. By integrating the techniques discussed, you maintain clean, manageable, and effective code structures.
No comments yet.