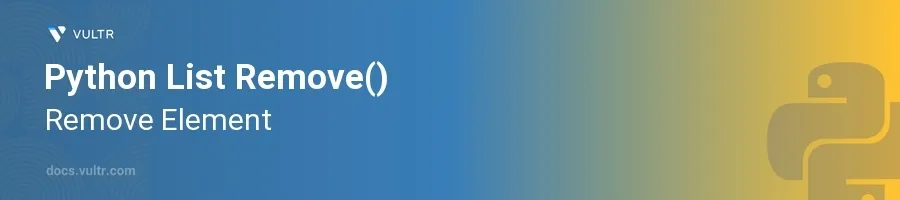
Introduction
The remove()
method in Python is a straightforward and efficient way to delete elements from a list. It modifies the list in place and targets the first occurrence of the specified element, which is an important behavior to remember when dealing with lists that contain duplicate elements. This method is ideal for situations where you know the exact value you want to remove but not necessarily its position in the list.
In this article, you will learn how to effectively utilize the remove()
method to handle various list operations in Python. The examples provided will demonstrate removing elements from simple lists, handling exceptions when elements are not found, and strategies for removing items in more complex scenarios.
Removing Elements from a List
Basic Element Removal
Start with a basic list containing some elements.
Apply the
remove()
method to delete a specific element.pythonfruits = ['apple', 'banana', 'cherry', 'banana'] fruits.remove('banana') print(fruits)
This code removes the first occurrence of
'banana'
from the listfruits
. After the operation, the list updates to include one less'banana'
.
Considerations with Duplicate Elements
Understand that
remove()
only deletes the first occurrence.pythonmulti_fruits = ['apple', 'banana', 'cherry', 'banana'] multi_fruits.remove('banana') multi_fruits.remove('banana') print(multi_fruits)
The method needs to be called as many times as there are occurrences of the element if the goal is to remove all instances.
Handling Exceptions When Element is Not Found
Using Try-Except Block
Prepare to catch the
ValueError
exception which raises when the element to remove does not exist in the list.pythonveggies = ['carrot', 'broccoli', 'cucumber'] try: veggies.remove('lettuce') except ValueError: print("Item not found in the list.")
When trying to remove
'lettuce'
, which is not in the listveggies
, Python throws aValueError
. The exception handling ensures the program continues running without crashing.
Strategies for Complex List Operations
Conditionally Removing Elements
Use a loop and conditionals to remove items under certain conditions.
pythonnumbers = [1, 2, 3, 4, 5, 6] for number in numbers[:]: if number % 2 == 0: numbers.remove(number) print(numbers)
This snippet removes all even numbers from the list
numbers
. Note the slicing used in the loop to create a copy of the list for iteration, avoiding modification issues during the loop.
Conclusion
The remove()
function in Python provides a reliable method to delete elements from a list based on their value. It is essential to handle exceptions properly when the target element might not be present in the list. For multiple occurrences, either repeat the method or use additional logic like loops with conditions. Mastering the remove()
method enhances your ability to manipulate lists efficiently in your Python projects, ensuring that data is modified accurately according to your program requirements.
No comments yet.