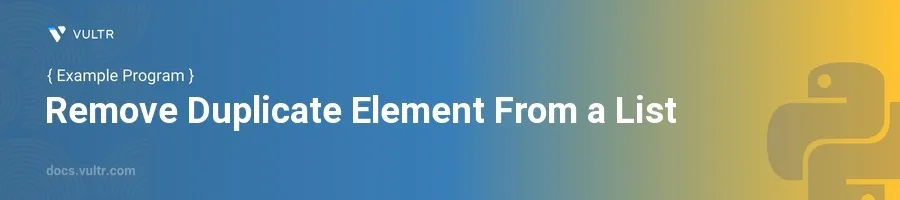
Introduction
Removing duplicates from a list is a common task in programming that involves creating a collection of items while ensuring each item is unique. This task comes in handy in data cleaning, processing, and when rules dictate uniqueness in a dataset. Effective handling of duplicates can lead to more efficient and error-free applications, particularly in Python, where lists are a versatile and widely used data structure.
In this article, you will learn how to remove duplicates from a list using different approaches in Python. The methods discussed include using a set, list comprehension, and a function from the itertools
module. By exploring examples of each method, you can determine which approach best suits your specific scenario.
Remove Duplicates Using a Set
Using a set is one of the most straightforward ways to remove duplicates from a list in Python because sets automatically discard any duplicate items.
Convert List to Set
Define a list with duplicate elements.
Convert the list to a set.
pythonoriginal_list = [1, 2, 2, 3, 3, 3, 4] unique_set = set(original_list)
Converting the list to a set removes any repeated elements, as sets do not allow duplicates.
Convert Set Back to List
Convert the set back to a list to restore it to its list form.
pythonrestored_list = list(unique_set) print(restored_list)
The output will display the unique elements of the original list in an undefined order, as sets do not maintain the order of elements.
Remove Duplicates Using List Comprehension
List comprehension offers a flexible way to create a new list by iterating over an existing one. This method is not only readable but also efficient for larger lists.
Create a New List with Unique Elements
Start with a list containing duplicates.
Use list comprehension to iterate through the list, adding elements only if they have not been added to the new list.
pythonoriginal_list = [1, 2, 2, 3, 3, 3, 4] unique_list = [] [unique_list.append(x) for x in original_list if x not in unique_list] print(unique_list)
This code snippet effectively iterates over each element and adds it to the
unique_list
only if it is not already present, thereby preserving order and removing duplicates.
Remove Duplicates Using Itertools
While itertools
does not directly provide a function to remove duplicates, you can leverage it alongside other Python features to create an efficient solution that also maintains the order of items.
Utilize itertools to Maintain Order
Import the
groupby
function fromitertools
.Use the function with a sort operation to maintain the list's order and remove duplicates.
pythonfrom itertools import groupby original_list = [1, 2, 2, 3, 3, 3, 4] sorted_list = sorted(original_list) # Sort before grouping unique_list = [key for key, group in groupby(sorted_list)] print(unique_list)
The
groupby
function groups the list by each unique element when the list is already sorted. The result is a list of unique elements in order.
Conclusion
In Python, numerous techniques exist to remove duplicates from a list, each having its own advantages depending on the situation. Using a set is the most straightforward method, ideal for cases where order is not important. List comprehension, on the other hand, offers flexibility and readability, maintaining the order and allowing for more complex conditions. Lastly, the itertools
groupby function serves well when order is essential and duplicates need to be efficiently removed post-sorting. Choose the method that best suits the nature of the data and the specific requirements of your project to effectively handle duplicates.
No comments yet.