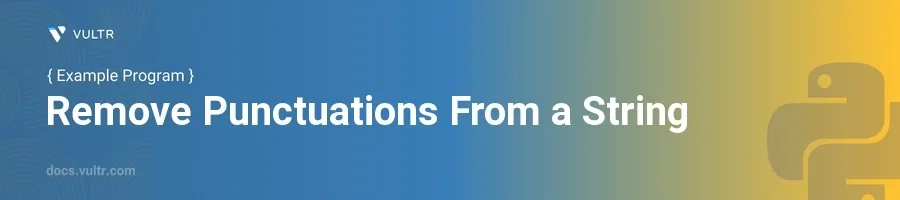
Introduction
Working with text data often involves cleaning and preprocessing steps, one of which is removing unnecessary punctuations. Punctuation marks can interfere with text analysis and processing tasks, such as natural language processing, data parsing, and more. Efficient removal of these characters from strings is crucial in a variety of programming scenarios.
In this article, you will learn how to effectively remove punctuation marks from strings in Python. Explore several methods, such as using Python's built-in string methods, regular expressions, and comprehension techniques. Each method will be clearly demonstrated through code examples to ensure you can apply these techniques in your own projects.
Using Python's String Methods
Remove Punctuation with str.replace()
Start with a string that contains punctuation.
Loop through the string and replace each punctuation mark with an empty string using
str.replace()
.pythonimport string def remove_punctuation(input_string): result = input_string for char in string.punctuation: result = result.replace(char, '') return result sample_text = "Hello, world! Python is fun; isn't it?" cleaned_text = remove_punctuation(sample_text) print(cleaned_text)
This function iterates over all common punctuation characters provided by the
string.punctuation
and replaces each occurrence with an empty string. The output for thesample_text
will be 'Hello world Python is fun isnt it'.
Using RegExp to Remove Punctuation
Import Python's
re
module for regular expressions.Define a pattern that matches all punctuation marks and replace them using
re.sub()
.pythonimport re import string def remove_punctuation_using_regex(input_string): regex_pattern = f"[{re.escape(string.punctuation)}]" result = re.sub(regex_pattern, "", input_string) return result sample_text = "Python? Yes, please! :)" cleaned_text = remove_punctuation_using_regex(sample_text) print(cleaned_text)
The
re.sub()
function substitutes all occurrences of the regex pattern (which includes all punctuation marks) with an empty string. The resulting output removes all punctuation, generating 'Python Yes please '.
Using List Comprehension
Efficient Punctuation Removal
Use a list comprehension to filter out punctuation marks from a string.
Convert the list back to a string using
str.join()
.pythonimport string def remove_punctuation_comprehension(input_string): result = ''.join([char for char in input_string if char not in string.punctuation]) return result sample_text = "Great work, everyone!" cleaned_text = remove_punctuation_comprehension(sample_text) print(cleaned_text)
The list comprehension checks every character in
input_string
and includes it in the result list if it is not a punctuation mark. Thejoin()
method then combines these characters back into a complete string without punctuation, resulting in 'Great work everyone'.
Conclusion
Removing punctuation from strings in Python can be handled efficiently through various methods, each suited to different needs and contexts. Whether you choose to implement a straightforward replace in a loop, leverage the power of regular expressions, or use a quick list comprehension, these techniques are fundamental in text preprocessing tasks. Adapt these methods to enhance your text handling capabilities in Python projects, ensuring your data is clean and ready for further analysis or processing.
No comments yet.